In This Topic
About Sorting
Sorting is the process of sorting the rows that reside on the same hierarchical level. The grid support multiple sorting rules that define a certain sorting order of the rows that reside at the same level in the grid grouping or self-referencing level.
Sorting rules are represented by instances of the NSortingRule class that reside in a NSortingRuleCollection accessible from the NGrid.SortingRules property. In most cases you will create filter rules that are associated with grid columns - the following code example first sorts by the Name and then by the Sales column:
Multiple Sorting Rules |
Copy Code
|
NSortingRule sortByName = new NSortingRule(grid.Columns.GetColumnByFieldName("Name"));
grid.SortingRules.Add(sortByName);
NSortingRule sortBySales = new NSortingRule(grid.Columns.GetColumnByFieldName("Sales"));
grid.SortingRules.Add(sortBySales);
|
Sorting can be visually performed by the user in several ways:
- Clicking on the Column Headers or Sorting Buttons - when the user clicks on the column headers or the sorting buttons, this will toggle the sorting direction in which that specific column is sorted. Additionally when the user holds down the Ctrl key, it is possible to perform sorting on multiple grid columns.
- Selecting the Sort Ascending or Sort Descending command from the Column Context Menu - the user can sort by a specific column by selecting the respective commands in the column context menu.
Sorting Rule and its Row Value and Sorting Direction
Each sorting rule has an assigned Row Value that associates each row with a specific value, by which the rule sorts the rows. The Row Value is specified by the RowValue property. Additionally each sorting rule has a sorting direction which is specified by the rule Sorting Direction property. The following code example creates a sorting rule and modifies its row value and sorting rule, so that the rule sorts the rows by the Name field in Descending order:
Sorting Rule Row Value and Sorting Direction |
Copy Code
|
NSortingRule sortByName = new NSortingRule();
sortByName.RowValue = new NFieldRowValue("Name");
sortByName.SortingDirection = ENSortingDirection.Ascending;
grid.SortingRules.Add(sortByName);
|
Sorting Rule and the associated Column
Each sorting rule can be associated with a grid column. The associated column is specified by the NSortingRule.Column property. There are several things, which happen when a sorting rule is associated with a column:
-
Default Row Value - there is a default row value associated with the sorting rule and it is the row value provided by the column. The following code example creates a sorting rule that sorts by the Total column:
Sort By Column |
Copy Code
|
// create a sorting rule that sorts by the Total column.
// Note that when the sorting rule is associated with a column, by default it sorts by it
// you can still however set the RowValue to alter the default column sorting, yet keep the Column association.
NSortingRule sortByTotal = new NSortingRule();
sortByTotal.Column = totalColumn;
grid.SortingRules.Add(sortByTotal);
|
-
Visual Interface - the visual interface will indicate that there is a sorting rule associated with the column in two ways:
Column Header Sorting Button |
Column Context Menu |
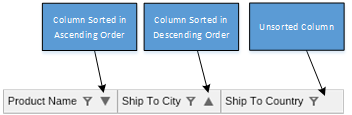 |
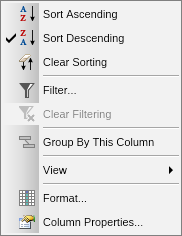 |
Sorting Rules created by Columns
When the user clicks on the sorting button of a column header, the grid asks the column to create a sorting rule for that column. By default the column creates a sorting rule that sorts by the column value (i.e. a sorting rule that is simply associated with the column). You may however want to create a custom sorting rule when the user clicks on the column header sorting button. This is achieved by handling the NColumn.CreateSortingRuleDelegate delegate, like shown in the following example:
Custom sorting rules created by columns |
Copy Code
|
column.CreateSortingRuleDelegate = delegate(NColumn theColumn)
{
// create a custom sorting rule
NSortingRule sortingRule = new NSortingRule();
NCustomRowValue<double> customRowValue = new NCustomRowValue<double>();
customRowValue.GetRowValueDelegate = delegate(NCustomRowValueGetRowValueArgs<double> args)
{
NDataSource dataSource = args.DataSource;
return (double)((double)dataSource[args.Row, "Price"] * (int)dataSource[args.Row, "Quantity"]);
};
sortingRule.RowValue = customRowValue;
return sortingRule;
};
|
See Also