Integrating NOV in ASP.NET Core MVC
In This Topic
Nevron Open Vision provides a .NET Standard 2.0 host, which can be easily integrated in an ASP.NET Core MVC project. This host doesn't depend on the operating system of the server, so it can be used on both Windows and Linux servers to generate images on the server. The only limitation is that currently it can only generate images in SVG format. The sections below discuss usage scenarios and provide sample code.
Generating Chart SVG Image on the Server
- Create a new ASP.NET Core MVC application.
- Right-click "Dependencies" in Solution Explorer, select "Add Project Reference", click the "Browse" button and add the following NOV DLLs as references:
- Nevron.Nov.Presentation
- Nevron.Nov.Host.Server
- Nevron.Nov.Text
- Nevron.Nov.Chart
- Initialize NOV in the entry point of the MVC application - the "Program.cs" by adding the following code right before the app.Run() call:
Initialize NOV |
Copy Code
|
// Apply your NOV license here. Skip this line of code while evaluating NOV
NLicenseManager.Instance.SetLicense("license-key");
// Initialize NOV
NNovApplicationInstaller.Install(
Nevron.Nov.Text.NTextModule.Instance,
Nevron.Nov.Chart.NChartModule.Instance
);
|
- Add the following action to the home controller - "Controllers\HomeController.cs". The chart view will not reside in a document and thus it won't have any styles to inherit. That's why an UI theme should be applied - see the code after the "Apply theme" comment below.
Generate a chart SVG image on the server |
Copy Code
|
public IActionResult GenerateSvgChart()
{
NSize chartSize = new(1000, 750);
// Create chart view
NChartView chartView = new NChartView();
chartView.Surface.CreatePredefinedChart(ENPredefinedChartType.Cartesian);
chartView.Surface.Size = chartSize;
// Apply theme
NWindows10Theme theme = new NWindows10Theme();
chartView.Document.StyleSheets.ApplyTheme(theme);
// Configure title
chartView.Surface.Titles[0].Text = "Programming Language Popularity";
// Configure chart
NCartesianChart chart = (NCartesianChart)chartView.Surface.Charts[0];
chart.SetPredefinedCartesianAxes(ENPredefinedCartesianAxis.XOrdinalYLinear);
// Add interlace stripe
NLinearScale linearScale = chart.Axes[ENCartesianAxis.PrimaryY].Scale as NLinearScale;
NScaleStrip strip = new NScaleStrip(new NColorFill(ENNamedColor.Beige), null, true, 0, 0, 1, 1);
strip.Interlaced = true;
linearScale.Strips.Add(strip);
// Create bar series
NBarSeries bar = new NBarSeries();
bar.LegendView.Mode = ENSeriesLegendMode.DataPoints;
bar.DataPoints.Add(new NBarDataPoint(18, "C++"));
bar.DataPoints.Add(new NBarDataPoint(15, "Ruby"));
bar.DataPoints.Add(new NBarDataPoint(21, "Python"));
bar.DataPoints.Add(new NBarDataPoint(23, "Java"));
bar.DataPoints.Add(new NBarDataPoint(27, "Javascript"));
bar.DataPoints.Add(new NBarDataPoint(29, "C#"));
bar.DataPoints.Add(new NBarDataPoint(26, "PHP"));
chart.Series.Add(bar);
// Export the chart to SVG in a memory stream
NChartVectorImageExporter exporter = new NChartVectorImageExporter(chartView.Content);
MemoryStream memoryStream = new MemoryStream();
exporter.SaveToStream(memoryStream, ENVectorImageFormat.Svg);
// Serve the memory stream to the client
memoryStream.Position = 0;
return File(memoryStream, SvgMimeType);
}
|
- Replace the markup of the "Views\Home\Index.cshtml" file with the following one:
MVC view that shows the chart SVG |
Copy Code
|
@{
ViewData["Title"] = "Home Page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
<p>The following SVG chart is generated by the .NET Standard 2.0 host of NOV on the server.
As a .NET Standard 2.0 library it doesn't depend on anything and can be used to generate server-side SVG charts on any platform - Windows and Linux.
</p>
<img src="@Url.Action("GenerateSvgChart", "Home")" />
</div>
|
The code above will generate the following web page:
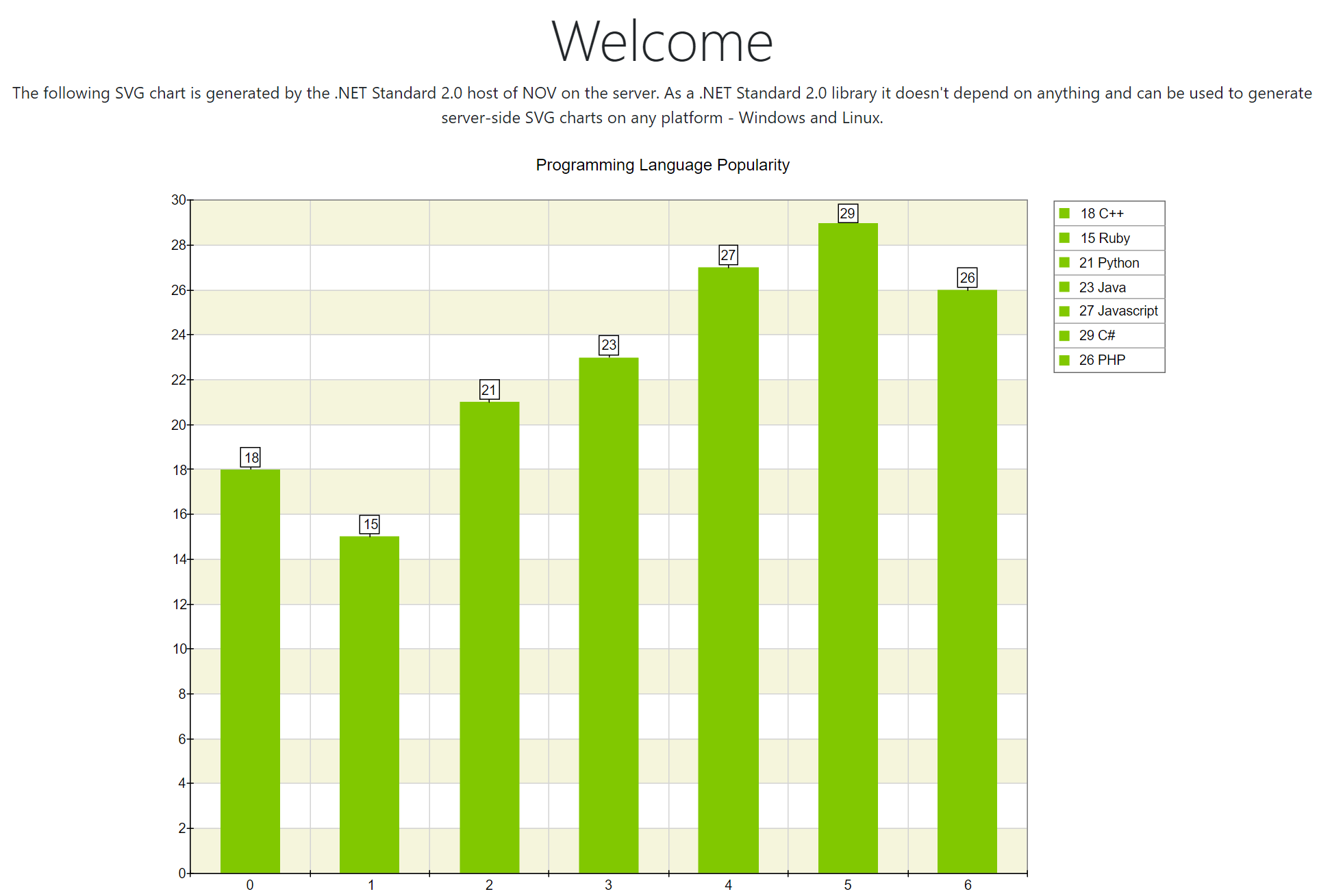
Generating Diagram SVG Image on the Server
- Create a new ASP.NET Core MVC application.
- Right-click "Dependencies" in Solution Explorer, select "Add Project Reference", click the "Browse" button and add the following NOV DLLs as references:
- Nevron.Nov.Presentation
- Nevron.Nov.Host.Server
- Nevron.Nov.Barcode
- Nevron.Nov.Text
- Nevron.Nov.Diagram
- Initialize NOV in the entry point of the MVC application - the "Program.cs" by adding the following code right before the app.Run() call:
Initialize NOV |
Copy Code
|
// Apply your NOV license here. Skip this line of code while evaluating NOV
NLicenseManager.Instance.SetLicense("license-key");
// Initialize NOV
NNovApplicationInstaller.Install(
Nevron.Nov.Barcode.NBarcodeModule.Instance,
Nevron.Nov.Text.NTextModule.Instance,
Nevron.Nov.Diagram.NDiagramModule.Instance
);
|
- Add the following action to the home controller - "Controllers\HomeController.cs". The drawing view will not reside in a document and thus it won't have any styles to inherit. That's why an UI theme should be applied - see the code after the "Apply theme" comment below.
Generate a diagram SVG Image on the server |
Copy Code
|
public IActionResult GenerateSvgDiagram()
{
NDrawingView drawingView = new NDrawingView();
// Apply theme
NWindows10Theme theme = new NWindows10Theme();
drawingView.Document.StyleSheets.ApplyTheme(theme);
// Create a simple diagram
NPage page = drawingView.ActivePage;
NBasicShapeFactory factory = new NBasicShapeFactory();
NShape shape1 = factory.CreateShape(ENBasicShape.Rectangle);
shape1.SetBounds(100, 100, 150, 100);
shape1.Text = "Rectangle";
page.Items.Add(shape1);
NShape shape2 = factory.CreateShape(ENBasicShape.Circle);
shape2.SetBounds(350, 250, 150, 100);
shape2.Text = "Ellipse";
page.Items.Add(shape2);
NRoutableConnector connector = new NRoutableConnector();
connector.UserClass = NDR.StyleSheetNameConnectors;
connector.Text = "Connector";
page.Items.Add(connector);
connector.GlueBeginToShape(shape1);
connector.GlueEndToShape(shape2);
// Export the diagram to SVG in a memory stream
NDrawingVectorImageExporter exporter = new NDrawingVectorImageExporter(drawingView.Content);
MemoryStream memoryStream = new MemoryStream();
exporter.SaveToStream(memoryStream, ENVectorImageFormat.Svg);
// Serve the memory stream to the client
memoryStream.Position = 0;
return File(memoryStream, SvgMimeType);
}
|
- Replace the markup of the "Views\Home\Index.cshtml" file with the following one:
MVC view that shows the diagram SVG |
Copy Code
|
@{
ViewData["Title"] = "Home Page";
}
<div class="text-center">
<h1 class="display-4">Welcome</h1>
<p>The following SVG diagram is generated by the .NET Standard 2.0 host of NOV on the server.
As a .NET Standard 2.0 library it doesn't depend on anything and can be used to generate server-side SVG charts on any platform - Windows and Linux.
</p>
<img src="@Url.Action("GenerateSvgDiagram", "Home")" />
</div>
|
The code above will generate the following web page:
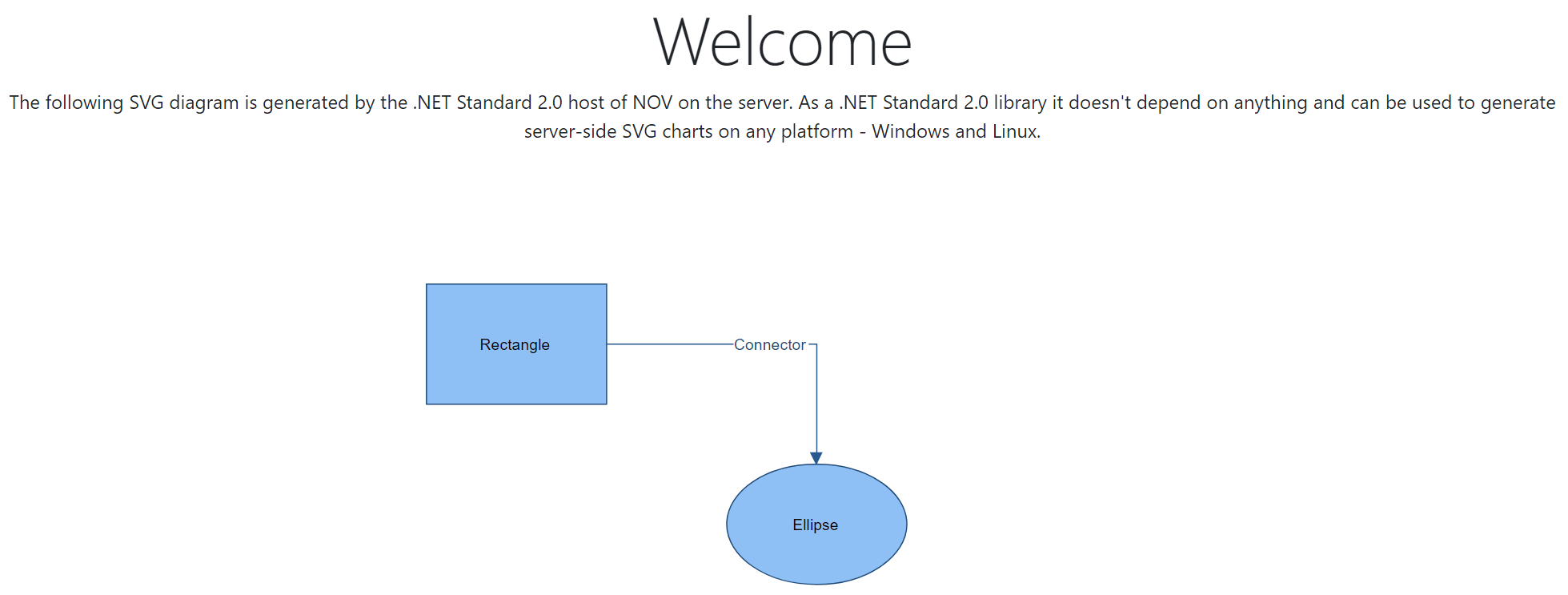
Generate SVG Image Source Code String
If it is required to only generate the source code of an SVG image as string, then the following piece of code can be used:
Generate SVG source code string |
Copy Code
|
NChartVectorImageExporter exporter = new NChartVectorImageExporter(chartView.Content);
NSvgDocument svgDocument = exporter.CreateSvg();
string svgSource = svgDocument.SaveToXml().SaveToString(false);
|
See Also