In This Topic
About Ports
Ports are connection points to which the begin and end points of 1D shapes can connect to. Ports are represented by the NPort class. The ports of a shape are contained in an instance of the NPortCollection class, which can be obtained from the Ports property of the shape.
Location and Direction
The location of the port is specified by the X and Y properties of NPort. If Relative is false, the X and Y properties specify the location of the port is shape local coordinates. If Relative is true, the X and Y properties specify the location of the port is shape local relative coordinates (i.e. relative to the shape Width / Height box). The GetLocation and SetLocation method get and set the port location in shape local coordinates (i.e. perform the relative to local conversion for you, if needed).
The direction of the port is defined by a pair of x and y coordinates specified by the DirX and DirY properties. The port direction is taken into account in routing and outward port connections, that is why it is important to specify correct directions for your ports.
The following example creates left, top, right and bottom ports for a shape:
Ports Example |
Copy Code
|
NShape shape = new NShape();
// ...
// left
NPort left = new NPort(0, 0.5d, true);
left.SetDirection(ENBoxDirection.Left);
shape.Ports.Add(left);
// top
NPort top = new NPort(0.5d, 0.0f, true);
top.SetDirection(ENBoxDirection.Up);
shape.Ports.Add(top);
// right
NPort right = new NPort(1.0d, 0.5d, true);
right.SetDirection(ENBoxDirection.Right);
shape.Ports.Add(right);
// bottom
NPort bottom = new NPort(0.5d, 1.0f, true);
bottom.SetDirection(ENBoxDirection.Down);
shape.Ports.Add(bottom);
|
FlowMode
The port FlowMode determines the type of end-points and/or other ports can connect to the port. It is a value from the ENPortFlowMode enumeration and can take the following values:
Input - input ports will allow to be connected only with end points of 1D shapes and output ports of 2D shapes.
Output - output ports will allow to be connected only with begin points of 1D shapes and input ports of 2D shapes.
InputOutput - the port is considered to be both input and output. This is the default value.
The helper properties of NPort - IsInput and IsOutput help you quickly determine whether the port is an input or output one.
GlueMode
The port GlueMode determines the type of glue that can be established with the port. It is a value from the ENPortGlueMode enumeration and can take the following values:
Inward - inward ports allow the glue of the begin and end points of 1D shapes and the glue of outward ports of 2D shapes. This is the default value.
Outward - an outward port is such a port that can glue to the inward ports of other shapes. When a shape with outward ports is moved close to a shape with inward ports, the shape with outward ports will get rotated and translated to match a pair of inward-outward ports locations and directions.
InwardOutward - the port is considered to be both inward and outward.
The helper properties of NPort - IsInward and IsOutward help you quickly determine whether the port is an inward or outward one.
The image below shows how the different types of ports look. The center port is a default port, which is a port with FlowMode = InputOutput and GlueMode = Inward.
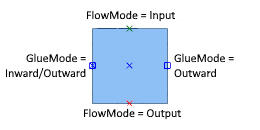