In This Topic
Nevron Schedule provides support for two types of appointments: one-time appointments and recurring appointments. Recurring appointments are appointments with a recurrence rule defined, which determines when the appointment occurs. The occurrences of a recurring appointment can easily be identified in the schedule by the small recurrence symbol placed at their top left corner. When the user tries to edit or delete an occurrence of a recurring appointment, the Nevron Schedule will show a dialog for selecting whether the operation should be applied to the recurring appointment (i.e. the whole series of occurrences) or only to the selected occurrence.
Nevron Schedule automatically generates the occurrences of a recurring appointment for the currently viewed date range. These occurrences reside in the Occurrences collection of the recurring appointment. You can browse this collection when needed, but do not modify it. It is automatically maintained and updated by Nevron.
Recurrence Rules
To make an appointment recurring, you should create a recurrence rule and assign it to the RecurrenceRule property of the appointment. Nevron Schedule provides support for different recurrence rules based on their frequency. The following diagram shows the recurrence rule hierarchy:
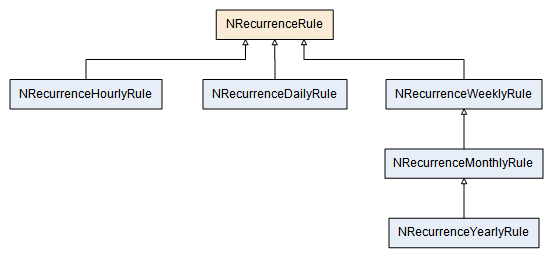
The base class of all recurrence rules NRecurrenceRule contains the following properties:
- Frequency - when overridden in a derived class, indicates the frequency of the recurrence rule instance. The currently supported recurrence rule frequencies are: hourly, daily, weekly, monthly and yearly.
- Interval - defines the interval of the recurrence. The unit of the interval depends on the recurrence rule frequency, for example, the interval unit for hourly rules is hour, for day rule is day, etc. By default set to 1.
- StartDate - defines the start of the recurrence range. This is the date and time after which the first occurrence of the recurring appointment happens. By default set to today.
- EndMode - determines the end mode of the recurrence. Can be Never, ByDate and AfterOccurrences. By default set to Never, which means that the recurrence continues indefinitely.
- EndDate - defines the end of the recurrence range. This is the date and time after which the recurring appointment does not occur any more. This property is taken into account only when EndMode is set to ByDate. By default set to 1 week from today.
- MaxOccurrences - specifies the number of times the recurring appointment occurs. This property is taken into account only when EndMode is set to AfterOccurrences. By default set to 10.
- ExcludedDates - a DOM array of DateTime objects, which specifies the dates and times excluded by this recurrence rule, i.e. the date and times occurrences for which will not be generated. By default not set, i.e. equal to null.
Code Examples
The following are a few examples of how to create and configure recurrence rules:
Recurrence Rule Examples |
Copy Code
|
// Create a rule, which occurs every 3 hours
NRecurrenceHourlyRule hourlyRule = new NRecurrenceHourlyRule();
hourlyRule.Interval = 3;
// Create a rule, which occurs every day
NRecurrenceDailyRule dailyRule = new NRecurrenceDailyRule();
// Create a rule, which occurs on the third day of every other month
// Use negative values for the last days of the month, for example -1 refers to the last day of the month
NRecurrenceMonthlyRule monthlyRule1 = new NRecurrenceMonthlyRule(3);
monthlyRule1.Interval = 2;
// Create a rule, which occurs on the last Sunday of every month
NRecurrenceMonthlyRule monthlyRule2 = new NRecurrenceMonthlyRule(ENDayOrdinal.Last, ENDayOfWeek.Sunday);
// Create a rule, which occurs on every May 7 and July 7, every year
NRecurrenceYearlyRule yearlyRule1 = new NRecurrenceYearlyRule(7, ENMonth.May | ENMonth.July);
// Create a rule, which occurs on the first Monday and Tuesday of May, June and August
NRecurrenceYearlyRule yearlyRule2 = new NRecurrenceYearlyRule(
ENDayOrdinal.First,
ENDayOfWeek.Monday | ENDayOfWeek.Tuesday,
ENMonth.May | ENMonth.June | ENMonth.August);
// Create a rule, which occurs every second day for one month from today
NRecurrenceDailyRule dailyRuleForMonth = new NRecurrenceDailyRule();
dailyRuleForMonth.EndMode = ENRecurrenceEndMode.ByDate;
dailyRuleForMonth.EndDate = DateTime.Today.AddMonths(1);
dailyRuleForMonth.Interval = 2;
|
Weekly and monthly rules use flag enum for specifying the day of week, so you can combine multiple enumeration values using the binary OR operator - '|'. The same goes for the yearly rule, which uses a flag enum for specifying the months in which the appointment should occur. This allows you to define very complex recurrence patterns when needed.
See Also