In This Topic
Each Cartesian Chart can have an unlimited number of X, Y, and Z (Depth) axes.
Creating a Cartesian Axis
You create a cartesian axis by creating an instance of the NCartesianAxis class and adding it to the Axes collection of a cartesian Chart:
C# |
Copy Code
|
NCartesianAxis xAxis = new NCartesianAxis();
cartesianChart.Axes.Add(xAxis);
NCartesianAxis yAxis = new NCartesianAxis();
cartesianChart.Axes.Add(yAxis);
|
The cartesian axis orientation and position are controlled by its anchor. There are two types of axis anchors – dock and cross axis anchors, which are discussed in the following sections.
Dock Axis Anchors
The cartesian dock axis is represented by the NDockCartesianAxisAnchor class. This anchor allows you to position the axis on one of the axis dock zones which are placed on the edges of the chart bounding box:
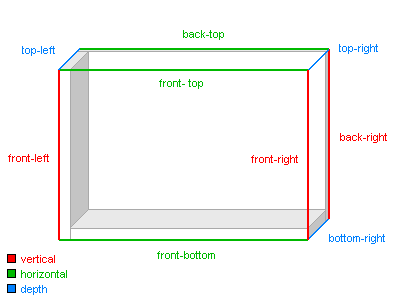
The following code shows how to anchor the x and y axes:
C# |
Copy Code
|
xAxis.Anchor = new NDockCartesianAxisAnchor(ENCartesianAxisDockZone.FrontBottom);
yAxis.Anchor = new NDockCartesianAxisAnchor(ENCartesianAxisDockZone.FrontLeft);
|
In 2D the chart uses only the FrontLeft, FrontTop, FrontRight, and FrontBottom axis zones. For convenience, the ENCartesianAxisDockZone enumeration also includes the alias names Left, Top, Right, and Bottom that refer to these axis zones.
Axis Dock Zone Levels
Each axis dock zone can contain one or more zone levels, and each level can contain one or more axes. The axes in one zone level cannot overlap axes in other zone levels in the same dock zone. Initially, axis dock zones do not contain any levels. When the first axis is placed in a particular zone it always creates a new level regardless of the setting specified by the CreateNewZoneLevel property of the anchor. Subsequent axes however can choose whether to share the zone level created by the previous axes or to create a new one that stacks on the previous zone level and its contained axes. The following code snippet shows how to create a Cartesian chart with three y axes where the third one is placed in a separate axis zone level:
C# |
Copy Code
|
NCartesianAxis yAxis1 = new NCartesianAxis();
NDockCartesianAxisAnchor dockAnchor1 = new NDockCartesianAxisAnchor();
dockAnchor1.DockZone = ENCartesianAxisDockZone.Left;
dockAnchor1.BeginPercent = 0;
dockAnchor1.EndPercent = 30;
yAxis1.Anchor = dockAnchor1;
cartesianChart.Axes.Add(yAxis1);
NCartesianAxis yAxis2 = new NCartesianAxis();
NDockCartesianAxisAnchor dockAnchor2 = new NDockCartesianAxisAnchor();
dockAnchor2.DockZone = ENCartesianAxisDockZone.Left;
dockAnchor2.BeginPercent = 70;
dockAnchor2.EndPercent = 100;
yAxis2.Anchor = dockAnchor2;
cartesianChart.Axes.Add(yAxis2);
NCartesianAxis yAxis3 = new NCartesianAxis();
NDockCartesianAxisAnchor dockAnchor3 = new NDockCartesianAxisAnchor();
dockAnchor3.CreateNewZoneLevel = true;
dockAnchor3.DockZone = ENCartesianAxisDockZone.Left;
dockAnchor3.BeginPercent = 30;
dockAnchor3.EndPercent = 70;
yAxis3.Anchor = dockAnchor3;
cartesianChart.Axes.Add(yAxis3);
|
Note that the code above specifies the amount of space occupied by the axis on the left chart plot edge - this is done through the BeghinPercent and EndPercent properties of the anchor.
Axes Dock Zone Levels Spacing
Each axis dock zone level has spacing applied before the previous level in the zone and spacing that should be applied after it when other levels are added. These spaces are controlled from the BeforeSpace and AfterSpace properties of the dock anchor.
Cross Axis Anchors
The cross axis anchor allows you to specify the axis orientation (Horizontal, Vertical, or Depth) and give the axis a reference point that it must cross. This reference point can be either a value on another axis of the chart (value crossing) or a point specified as an offset from the begin, end, or center position on another axis (model crossing).
Axis Value Crossing
To create an axis that crosses another axis at a certain logical value you need to assign an instance of the NCrossCartesianAxisAnchor class to its Anchor property. The NCrossCartesianAxisAnchor Crossing property must be set to an instance of the NValueAxisCrossing class, that specifies an axis and a logical value at which the crossing must occur.
The following code creates a chart with an X and Y axes that cross at 0:
C# |
Copy Code
|
// add the x and y axes
NCartesianAxis xAxis = new NCartesianAxis();
cartesianChart.Axes.Add(xAxis);
NCartesianAxis yAxis = new NCartesianAxis();
cartesianChart.Axes.Add(yAxis);
// cross them
NCrossCartesianAxisAnchor yCross = new NCrossCartesianAxisAnchor(ENCartesianAxisOrientation.Vertical, ENScaleOrientation.Left, 0, 100);
yCross.XAxisCrossing = new NValueAxisCrossing(xAxis, 0);
yAxis.Anchor = yCross;
NCrossCartesianAxisAnchor xCross = new NCrossCartesianAxisAnchor(ENCartesianAxisOrientation.Horizontal, ENScaleOrientation.Right, 0, 100);
xCross.YAxisCrossing = new NValueAxisCrossing(yAxis, 0);
xAxis.Anchor = xCross;
|
Axis Model Crossing
Similarly, to create an axis that crosses another axis on a certain model value you need to assign an instance of the NCrossCartesianAxisAnchor class to its Anchor property, but in this case, the Crossing property must be set to an instance of the NModelAxisCrossing class, that accepts a reference axis, model value and alignment relative to the reference axis:
C# |
Copy Code
|
// add the x and y axes
NCartesianAxis xAxis = new NCartesianAxis();
cartesianChart.Axes.Add(xAxis);
NCartesianAxis yAxis = new NCartesianAxis();
cartesianChart.Axes.Add(yAxis);
// cross them
NCrossCartesianAxisAnchor yCross = new NCrossCartesianAxisAnchor(ENCartesianAxisOrientation.Vertical, ENScaleOrientation.Left, 0, 100);
yCross.XAxisCrossing = new NModelAxisCrossing(xAxis, 0, ENAxisCrossAlignment.Center);
yAxis.Anchor = yCross;
NCrossCartesianAxisAnchor xCross = new NCrossCartesianAxisAnchor(ENCartesianAxisOrientation.Horizontal, ENScaleOrientation.Right, 0, 100);
xCross.YAxisCrossing = new NModelAxisCrossing(yAxis, 0, ENAxisCrossAlignment.Center);
xAxis.Anchor = xCross;
|
See Also