In This Topic
Designers are used to provide editors for node children and members. When you want to define a specific editor in the designer, you are typically going to use an instance of the NEditorTemplate class, which is essentially a pair of an editor type and settings for this editor (see Editor Templates for more info).
Following is an overview of the editors that are defined by the designer.
Standalone and Embedded Editors
The Standalone Editor Template defines the editor which is created for the node, when the node is the root of the edited hierarchy. It is specified by the StandaloneEditorTemplate property. If an standalone editor template is not specified, the returned default template depends on the node schema type. If the node is a leaf node (does not have any child nodes), the NNodeMembersEditor.DefaultEditorTemplate is returned, otherwise the NNodeSubtreeEditor.DefaultTemplate is returned.
The Embedded Editor Template is the editor which is created, when the node is embedded in the hierarchy editor of another node. By default this is the NNodeMembersEditor.DefaultEditorTemplate.
The following image illustrates a Standalone Editor created for a NOV Diagram Shape, that aggregates an Embedded Editor for a control point:
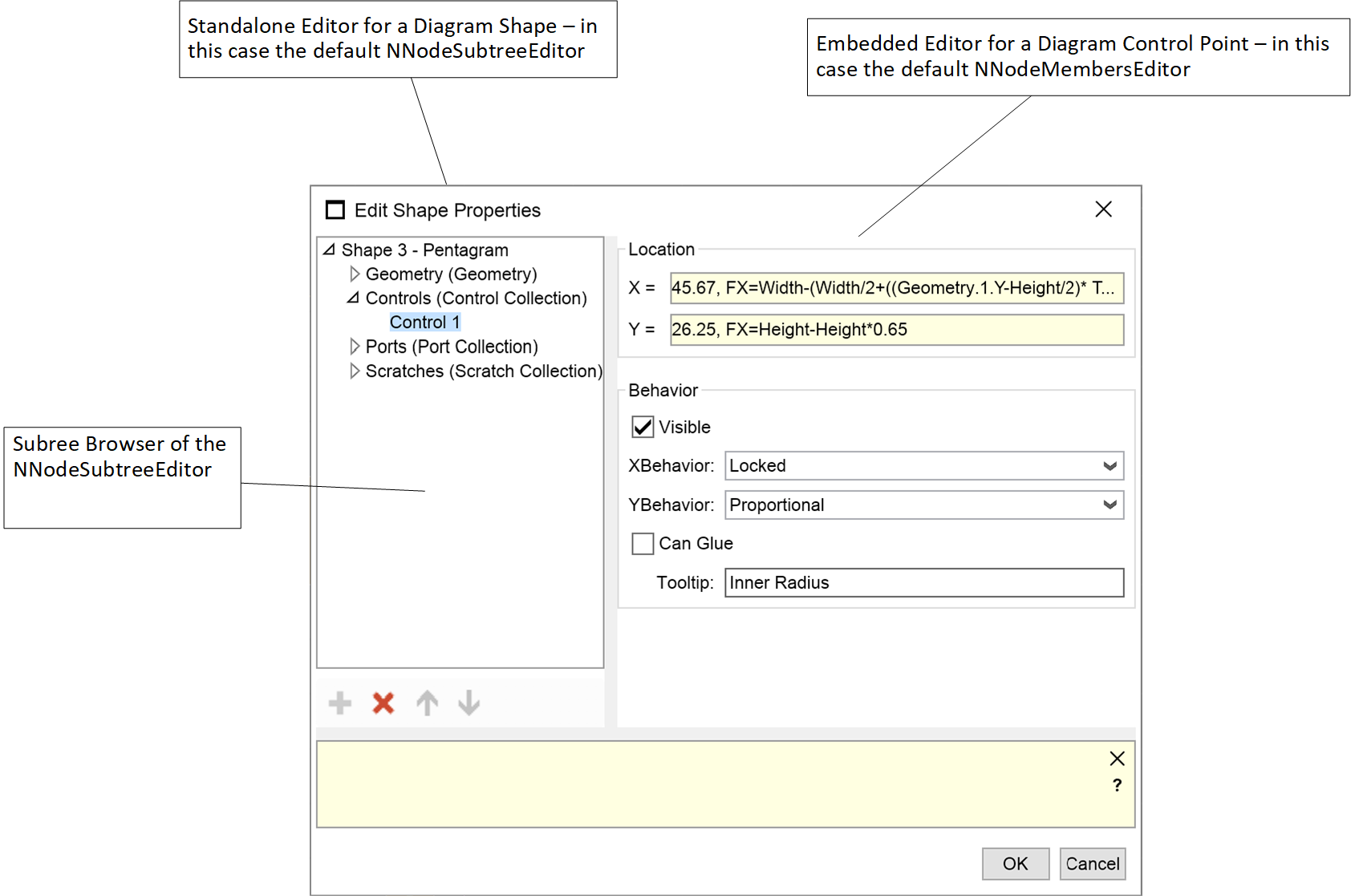
fig 1. Standalone Editor integrating the Embedded Editor of another node
See General Editors for more information.
Members Browsability
Node schemas can generally contain two types of members - properties and child slots. For both of these member types, the designer defines their browsability - i.e. whether the specific member should be displayed or not.
The NDesigner - GetMemberBrowsable and SetMemberBrowsable methods help you control the visibility of a certain member inside the NNodeMembersEditor (an editor that aggregates the members of a node).
By default properties are browsable (controlled by the DefaultPropertyBrowseMode property), while child slots are not (controlled by the DefaultContainerChildrenBrowseMode property). You can hide certain properties from editing by setting them as non-browsable like this:
Hiding Properties from Editors |
Copy Code
|
public class MyNode : NNode
{
...
public static readonly NProperty MyProperty;
public class MyNodeDesigner : NDesigner
{
public MyNodeDesigner()
{
SetMemberBrowsable(MyNode.MyProperty, false);
}
}
}
|
Child slots are by default not browsable and will only appear in the hierarchy browser of hierarchy editors. Whether or not the child slots of the node are by default visible in the hierarchy browsers is controlled by the DefaultChildSlotHierachyBrowseMode property. You can hide certain child slots from editing by setting them as non-hierarchy browsable like this:
Hiding Child Slots from Hierarchy Browsing |
Copy Code
|
public class MyNode : NNode
{
...
public static readonly NChild MyChild;
public class MyNodeDesigner : NDesigner
{
public MyNodeDesigner()
{
SetChildHierarchyBrowsable(MyNode.MyChild, false);
}
}
}
|
Members Editors
The type of editors created for the different node members (properties and child slots), by default depends on their type. For properties the editor created depends on their type, for child slots the editor is by default the NEmbeddedChildEditor, which aggregates the Standalone Editor of the node.
The following table summarizes the property editors for the different CLR types:
Type |
Property Editor |
Object |
NDefaultPropertyEditor |
Boolean |
NBooleanPropertyEditor |
String |
NStringPropertyEditor |
Double |
NDoublePropertyEditor |
Single |
NSinglePropertyEditor |
Int32 |
NInt32PropertyEditor |
UInt32 |
NUInt32PropertyEditor |
Int64 |
NInt64PropertyEditor |
Char |
NCharPropertyEditor |
Enums |
NEnumPropertyEditor |
DateTime |
NDateTimePropertyEditor |
TimeSpan |
NTimeSpanPropertyEditor |
You may be required to change the editor for certain properties - you do that with the help of the SetMemberEditorTemplate method.
For example, suppose that you have a double property you may want the user to only be able to enter values in the [0-100] range with a step of 0.1 and 2 digits precision, you can do that by specifying an editor template for the property, that has a modified properties like shown in the following example:
Setting an custom editor template for a property |
Copy Code
|
public class MyNode : NNode
{
...
public static readonly NProperty MyDoubleProperty;
public class MyNodeDesigner : NDesigner
{
public MyNodeDesigner()
{
// create a custom editor template
NEditorTemplate myTemplate = new NEditorTemplate(typeof(NDoublePropertyEditor));
myTemplate.EditorProperties[NDoublePropertyEditor.MinimumProperty] = -100.00;
myTemplate.EditorProperties[NDoublePropertyEditor.MaximumProperty] = 100.00;
myTemplate.EditorProperties[NDoublePropertyEditor.StepProperty] = 0.1;
myTemplate.EditorProperties[NDoublePropertyEditor.DecimalPlacesProperty] = 2;
SetMemberEditorTemplate(MyDoubleProperty, myTemplate);
}
}
}
|
Many of the editor types contain predefined editor templates, that you can use. Explore the Programmers Reference for each editor type to check for static members with Template suffix - these are predefined editor templates with certain property modifications.