The radar chart (also known as spider or star chart) is a two-dimensional chart that shows the relationship between three or more variables represented on axes starting from the same point. Radar charts are commonly used when the user has to compare data consisting of several categories, that are not related to each other. The following image shows a typical radar chart:
To create a radar chart you need to create an instance of the NRadarChart type and add it to the chart surface. The following code shows how to create a radar chart containing several data points:
C# |
Copy Code
|
// Add a NOV widget inside the form
NChartView chartView = new NChartView();
// create a dock panel with a label and chart
NDockPanel dockPanel = new NDockPanel();
chartView.Surface.Content = dockPanel;
// create a docked label
NLabel label = new NLabel();
label.Text = "My First Radar Chart";
label.Margins = new NMargins(10);
label.Font = new NFont(NFontDescriptor.DefaultSansFamilyName, 12);
label.TextFill = new NColorFill(NColor.Black);
label.TextAlignment = ENContentAlignment.MiddleCenter;
NDockLayout.SetDockArea(label, ENDockArea.Top);
dockPanel.AddChild(label);
// create a new polar chart
NRadarChart radarChart = new NRadarChart();
radarChart.Margins = new NMargins(10);
NDockLayout.SetDockArea(radarChart, ENDockArea.Center);
dockPanel.AddChild(radarChart);
AddAxis(radarChart, "Vitamin A");
AddAxis(radarChart, "Vitamin B");
AddAxis(radarChart, "Vitamin C");
AddAxis(radarChart, "Vitamin D");
// create a radar area series
NRadarAreaSeries radarSeries = new NRadarAreaSeries();
radarSeries.DataPoints.Add(new NRadarAreaDataPoint(20));
radarSeries.DataPoints.Add(new NRadarAreaDataPoint(80));
radarSeries.DataPoints.Add(new NRadarAreaDataPoint(34));
radarSeries.DataPoints.Add(new NRadarAreaDataPoint(79));
radarChart.Series.Add(radarSeries);
|
There are two types of radar charts - single and multi-measure. Single measure radar charts are used when you want to compare values with common measurement and magnitude (for example the chart created by the above code is a single measure radar as the vitamins are compared on the same scale that ranges from 0 to 100). The following image shows how the single measure radar chart looks like:
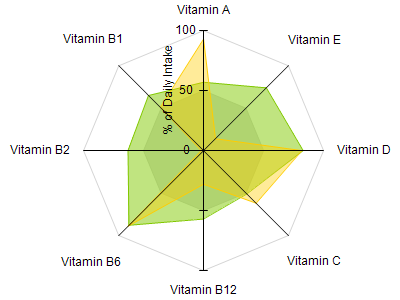
Multi measure radar charts on the other hand are used when you want to compare values that differ in magnitude and/or measure. By default when you create a radar chart it will be configured as single measure radar. To switch to multi measure radar chart you must set the RadarMode property to ENRadarMode.MultiMeasure. The following code snippet shows how to do that:
C# |
Copy Code
|
radarChart.RadarMode = ENRadarMode.MultiMeasure;
|
The following image shows a multi-measure radar chart that compares several counties along different measurements such as population, water, land, etc:

Each radar axis can be annotated with a text label. This label can appear on the outer side of the radar rim and there are various properties you can control to modify its appearance and alignment. This section describes those properties and how they work together.
Title Angle
The radar axis title angle is controlled from the TitleAngle property, which accepts an object of type NScaleLabelAngle. The following code snippet applies horizontal orientation to the radar label:
C# |
Copy Code
|
NRadarAxis radarAxis = new NRadarAxis();
radarAxis.Title = "Radar Axis";
radarAxis.TitleAngle = new NScaleLabelAngle(ENScaleLabelAngleMode.View, 0);
radarChart.Axes.Add(radarAxis);
|
Title Offset
The title offset controls the distance between the radar axis title and the outer radar rim. Increasing this value will move the radar axis titles farther from the radar plot - the following code snippet applied a distance of 20 dips between the radar rim and the title:
C# |
Copy Code
|
NRadarAxis radarAxis = new NRadarAxis();
radarAxis.Title = "Radar Axis";
radarAxis.TitleAngle = new NScaleLabelAngle(ENScaleLabelAngleMode.View, 0);
radarAxis.TitleOffset = 20;
radarChart.Axes.Add(radarAxis);
|
Title Position Mode
The title position mode specifies how the title is positioned relative to the ray defined from the center of the radar chart and the radar axis. There are two options:
ENRadarTitlePositionMode |
Description |
Center |
The title is positioned so that its center point lies on the radar axis vector (this is the default) |
NearestPoint |
The title is positioned so that the nearest point of the title that does not touch the radar rim lies on the radar axis vector |
The following pictures show how the labels will be positioned depending on the value of the TitlePositionMode property:
ENRadarTitlePositionMode.Center |
ENRadarTitlePositionMode.NearestPoint |
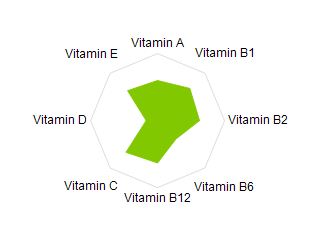 |
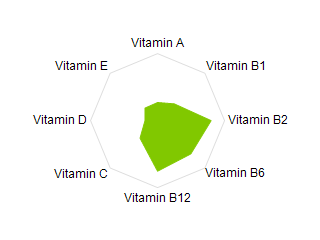 |
The following code snippet modifies the radar title position mode:
C# |
Copy Code
|
NRadarAxis radarAxis = new NRadarAxis();
radarAxis.Title = "Radar Axis";
radarAxis.TitlePositionMode = ENRadarTitlePositionMode.NearestPoint;
radarChart.Axes.Add(radarAxis);
|
Title Text Alignment
When you have multi line radar axis titles it is sometimes necessary to specify left, right, or center alignment depending on the position of the title relative to the radar center. This is achieved by setting the TitleAutomaticAlignment property to true:
C# |
Copy Code
|
radarAxis.TitleAutomaticAlignment = true;
|
The following picture shows the effect of this property:
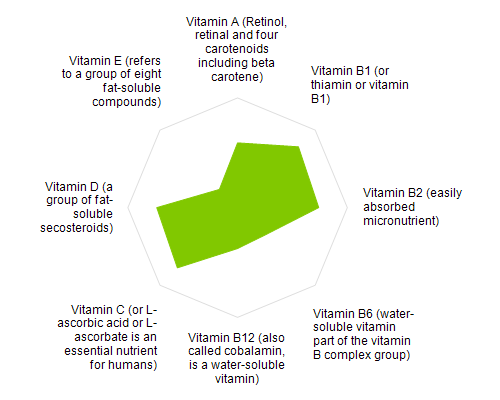