In This Topic
Raster images are made up of a set grid of dots called pixels where each pixel is assigned a color value. This makes raster images resolution dependent and their quality deteriorates when they are scaled up or down.
Image Formats
Image formats are represented by the NImageFormat type. The framework supports the following commonly used formats for image interchange and presentation:
-
Raster image formats
- PNG (Portable Network Graphics) – encoding and decoding.
- JPEG (Joint Photographic Experts Group) – encoding and decoding.
- GIF (Graphics Interchange Format) – decoding only.
- BMP (Windows Bitmap) – encoding and decoding.
-
Vector image formats
- SVG (Scalable Vector Graphics) - encoding and decoding.
- WMF (Windows Metafile) - decoding only.
- EMF (Enhanced Metafile) - decoding only.
NOV’s imaging library provides its own implementations of the encoders and decoders listed above, so that the functionality is available on all supported platforms. Furthermore, the library can use platform-provided image codecs where they are available.
The built-in formats can be accessed through static fields of NImageFormat – for example NImageFormat.Jpeg denotes the JPEG format, NImageFormat.Png – the PNG format, etc.
It is possible to register a custom image format using the NImageFormat.CreateFormat method. The method requires an instance of a type that implements the INImageFormatProvider interface.
Besides the common formats, the framework also offers its own image format called Nevron Raster Image (NRI). An NRI file consists of a signature, followed by a file header with basic image information and the pixel data compressed using the Zlib compression algorithm. The NRI image format is described in the table below:
Signature |
3 bytes (UTF-8 encoded "NRI") |
Width |
4 bytes, Int32 |
Height |
4 bytes, Int32 |
Resolution |
4 bytes, Single |
PixelFormat |
1 byte |
Pixel Data |
Zlib compressed (Stride * Height) bytes, where Stride is the number of bytes per row, aligned to 4 bytes |
Creating Images
You can create images by using one of the constructors of the NImage class.
Alternatively you can use one of the following static methods of the NImage class:
- NImage.FromFileEmbedded - creates an image from the file with a given full file path or from a given file object. The file content is read and embedded into the created image, so it does not depend on the file. It is recommended to embed image files, because this makes your documents portable - everything is included into the document and there's no need to distribute additional files with it.
- NImage.FromLocalFileEmbedded - same as the above, but the image is loaded synchronously.
- NImage.FromFileLinked - creates an image from the file with a given full file path or from a given file object. The image is linked to that file, which means that the image depends on the file. If the file gets changed, the image will be updated, but if the file is deleted the image won't work properly anymore.
- NImage.FromLocalFileLinked - same as the above, but the image is loaded synchronously.
- NImage.FromStream - creates an image from a given stream. The stream is read as a byte array and then an image is created from this byte array, so the image does not depend on the stream.
- NImage.FromRaster - creates an image, which aggregates a raster.
- NImage.FromResource - creates an image from an embedded resource.
- NImage.FromBytes - creates an image from a byte array.
- NImage.FromUri - creates and image from a file or an HTTP/HTTPS URI.
- NImage.FromDataUriString - creates an image from a data uri (Base64 encoded) string.
Full file paths and linking images to files to images are not supported on sandboxed platforms like Blazor WebAssembly and Mac Store applications.
You can also create raster images by passing an image source to the corresponding NImage constructor. For more information take a look at the Image Sources section at the end of this topic.
Displaying Images
NImage is an attribute type that represents images within the Document Object Model. It acts as a wrapper around NImageSource that helps for the integration of image sources inside the DOM.
Each NImage object contains an NImageSource instance that is assigned at construction time. Most of the NImage constructors create this instance internally, but it is also possible to specify an existing NImageSource instance. The following example demonstrates the initialization of an NImage object with a file image source:
Creating an NImage object |
Copy Code
|
NImage image = NImage.FromFileEmbedded(@"D:\TestImage.png");
|
The newly created NImage object can be assigned as an attribute to a DOM node. For example, the image box widget (NImageBox) has a property of type NImage, which specifies the displayed image:
Changing the image of an ImageBox widget |
Copy Code
|
NImageBox imageBox = new NImageBox();
imageBox.Image = image;
|
You can also use an image to create an image fill, which can then be used to fill an arbitrary widget. The following example fills a stack panel with an image:
Filling a widget with an image |
Copy Code
|
NImage image = NImage.FromFileEmbedded(@"D:\flower.png");
stackPanel.BackgroundFill = new NImageFill(image);
|
You can use the
TextureMapping property of the image box or the image fill to specify how the image should be rendered in the available area.
Image Sources
The sources of image data used in an application can vary from embedded resources and in-memory buffers to local files and web images. To ensure that all these types of sources can be used transparently with the framework graphics and the DOM, the NOV framework provides a set of types to represent images with different physical location and provide convenient access to them. The base type for all image sources is called NImageSource. The following table displays the hierarchy of the most commonly used image source types:
Type |
Description |
NImageSource |
Abstract base type for all image sources. |
|
NRasterImageSource |
The image source is an NRaster object. |
|
NEncodedImageSource |
Abstract base type for image sources that provide encoded image data. |
|
NBytesImageSource |
The image source is a byte array containing encoded image data. |
|
NEmbeddedResourceImageSource |
The image source is an embedded resource containing encoded image data. |
|
NUriImageSource |
Abstract base class for image sources the location of which is specified with an URI |
|
NFileImageSource |
The image source is a local or network file. |
|
NHttpImageSource |
The image source is a file accessible through HTTP. |
Following are several examples that demonstrate how image sources are created and used. The code below paints a few rectangles in a raster object and creates an NRasterImageSource from it:
Creating a Raster Image Source |
Copy Code
|
NRaster raster = new NRaster(100, 60, NPixelFormat.ARGB32);
raster.Clear(NColor.OliveDrab);
raster.FillRect(NColor.Crimson, new NRectangleI(10, 10, 70, 30));
raster.FillRect(NColor.Orange, new NRectangleI(20, 20, 70, 30));
NRasterImageSource rasterImgSrc = new NRasterImageSource(raster);
|
The generated image can be painted on the screen or in another rendering context using an NPaintVisitor object:
Drawing an image |
Copy Code
|
visitor.PaintImage(rasterImgSrc, new NPoint(10, 10));
|
The result is displayed below:
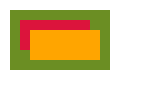
Any other type of image source can be rendered the same way. For example, for an image contained in a local file the following code can be used:
Drawing an image from a File Image Source |
Copy Code
|
NFileImageSource fileImgSrc = new NFileImageSource(NFileSystem.GetFile(@"D:\TestImage.png"), true);
...
visitor.PaintImage(fileImgSrc, new NPoint(10, 10));
|
When dealing with remotely located resources the data does not become available instantly – there is a period of time during which the data is traveling towards the recipient. During this period the image source object can still be used, but it renders an empty image. When the actual image data arrives, the image source raises the ImageSourceChanged event. This notification can be used to initiate a repaint of the widget that displays the image source.
All types derived from NImageSource implement common functionality that facilitates the access to the image information. In general all image sources are able to:
- Provide basic information like image width, height and resolution (see Width, Height, Resolution and ImageInfo properties).
- Create an NRaster object that represents the image (see CreateRaster and TryCreateRaster methods).
- Create an NImageData object that contains the image in encoded form (see GetEncodedData method).
- Report whether all pixels have the same alpha value and report the uniform alpha value itself (see GetUniformAlpha method).
For image sources with multiple frames it is possible to obtain the number of frames, as well as information about each particular frame. Please refer to NImageInfo.FrameCount and NImageInfo.GetFrameInfo for details.
Multi-frame images can also be animated. For more information head to the Raster Image Animation topic.
See Also