NOV Diagram supports automatic connector splitting when the user drops a 2D shape over a 1D shape.
Connector splitting is enabled by default and works in the following way:
- The user moves a 2D shape (the circle in the image below) over a connector.
- The connector is highlighted to inform the user that it will get split if he or she drops the 2D shape over it.

- The user drops the shape over the connector.
- The connector gets split to 2 connectors by the dropped shape.

You can enable or disable connector splitting in several ways:
- To enable/disable connector splitting for the whole page use the Enable1DShapeSplitting property of the page interactor:
Disable connector splitting for the whole page |
Copy Code
|
page.Interaction.Enable1DShapeSplitting = false;
|
- To enalble/disable connector splitting for a specific connector, use its Splittable property:
Disable splitting of a specific connector |
Copy Code
|
connector.Splittable = false;
|
- To enable/disable a 2D shape from splitting connectors, use its CanSplit property:
Disable a 2D shape from splitting connectors |
Copy Code
|
shape.CanSplit = false;
|
To split a connector programmatically you can use the Split method of NShape and pass a 2D shape to split the connector with. The split operation will succeed only if the connector is added to a page, its Splittable property it true and the CanSplit property of the passed 2D shape is true.
The following is an example of a connector split with a 2D shape (the small circle at the middle of the connector):
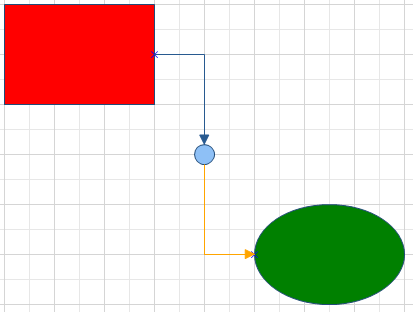
The sample diagram above is created with the following piece of code (the connector splitting is the second to last line of code):
Connector splitting |
Copy Code
|
NPage page = drawingView.ActivePage;
NBasicShapeFactory factory = new NBasicShapeFactory();
// Create the first shape
NShape shape1 = factory.CreateShape(ENBasicShape.Rectangle);
shape1.SetBounds(100, 100, 150, 100);
shape1.Geometry.Fill = new NColorFill(NColor.Red);
page.Items.Add(shape1);
shape1.Ports.Clear();
NPort port1 = new NPort(1, 0.5, true);
port1.SetDirection(ENBoxDirection.Right);
shape1.Ports.Add(port1);
// Create the second shape
NShape shape2 = factory.CreateShape(ENBasicShape.Circle);
shape2.SetBounds(350, 300, 150, 100);
shape2.Geometry.Fill = new NColorFill(NColor.Green);
page.Items.Add(shape2);
shape2.Ports.Clear();
NPort port2 = new NPort(0, 0.5, true);
port2.SetDirection(ENBoxDirection.Left);
shape2.Ports.Add(port2);
// Connect the shapes with a routable connector
NRoutableConnector connector = new NRoutableConnector();
connector.RerouteMode = ENRoutableConnectorRerouteMode.WhenNeeded;
connector.UserClass = NDR.StyleSheetNameConnectors;
page.Items.Add(connector);
connector.GlueBeginToPort(port1);
connector.GlueEndToPort(port2);
// Evaluate the drawing document to calculate coordinates
drawingView.Document.Evaluate();
// Get the middle point of the middle segment of the connector
int segmentCount = connector.Model.SegmentsCount;
NPoint middlePoint = connector.Model.GetSegmentMidPoint(segmentCount / 2);
// Create a 2D shape for split shape and place it at the connector middle point
NShape splitShape = new NShape();
splitShape.SetPinPoint(middlePoint);
{
// Set shape geometry and size.
// Remove the following 3 lines of code to get an invisible 2D shape.
splitShape.Geometry.AddRelative(new NDrawEllipse(0, 0, 1, 1));
splitShape.Width = 20;
splitShape.Height = 20;
}
page.Items.Add(splitShape);
// Split the connector with the split shape
NRoutableConnector newConnector = (NRoutableConnector)connector.Split(splitShape);
// Make the new connector orange
newConnector.Geometry.Stroke = new NStroke(1, NColor.Orange);
|