In This Topic
NOV Diagram comes with a family tree shapes library, which makes it easy to create family tree diagrams.
Family Tree Library
The family tree shapes library is located in the following subfolder in the installation folder of NOV:
- Resources\ShapeLibraries\Family Tree\Family Tree Shapes.nlb
It is best to load the file format library using the GEDCOM file format's helper method in the entry point of the application. Thus you will have to load it only once, for example in the entry point of the application or right before creating the first family tree diagram.
Like most file operations in NOV, loading of the family tree library from file is an asynchronous operation, so the Then method of the returned promise should be used to get notified when the family tree library has been loaded.
The code below demonstrates how to load the family tree library:
Load the family tree shapes library |
Copy Code
|
// Load the GEDCOM file format's family tree library (this needs to be done only once)
NFile libraryFile = NApplication.ResourcesFolder.GetFile(NPath.Current.Combine(
"ShapeLibraries", "Family Tree", "Family Tree Shapes.nlb"));
NDrawingFormat.Gedcom.LoadFamilyTreeLibraryFromFileAsync(libraryFile).Then(
delegate (NUndefined ud)
{
// Family tree library loaded successfully, so create the family tree diagram
CreateFamilyTree(drawingView.ActivePage);
},
delegate (Exception ex)
{
// Failed to load the family tree library
}
);
|
The family tree shape library should be distributed (as a separate file or an embedded resource) with any NOV application that needs to import GEDCOM files or to create family tree diagrams. To load the library, the async LoadFamilyTreeLibraryFromFileAsync or the sync LoadFamilyTreeLibrary from stream methods of the GEDCOM file format should be used.
Family Tree Shapes
After the family tree library has been loaded, it can be used to create family tree shapes with the following sample method:
Method for creating family tree shapes |
Copy Code
|
private NShape CreateShape(ENFamilyTreeShape familyShape)
{
NLibraryItem libraryItem = NDrawingFormat.Gedcom.FamilyTreeLibrary.Items[(int)familyShape];
NShape shape = (NShape)libraryItem.Items[0];
return (NShape)shape.DeepClone();
}
|
There are 2 family tree shapes
-
Person (Male and Female) shape - a rectangular 2D shape that shows information about a person: name, dates, places, photo, etc. The person shape is blue if it represents a male and pink if it represents a female. The following shape properties are supported by person shapes:
- Gender
- FirstName
- LastName
- MarriedName
- Email
- Photo
- BirthDate
- BirthPlace
- DeathDate
- DeathPlace
-
Relationship shape - a circular 2D shape that shows information for a relationship: dates, places, etc. The relationship shape is green when no dates are specified for it, blue if only marriage date is specified and red if both marriage date and divorce dates are specified. The following shape properties are supported by relationship shapes:
- MarriageDate
- MarriagePlace
- DivorceDate
- DivorcePlace
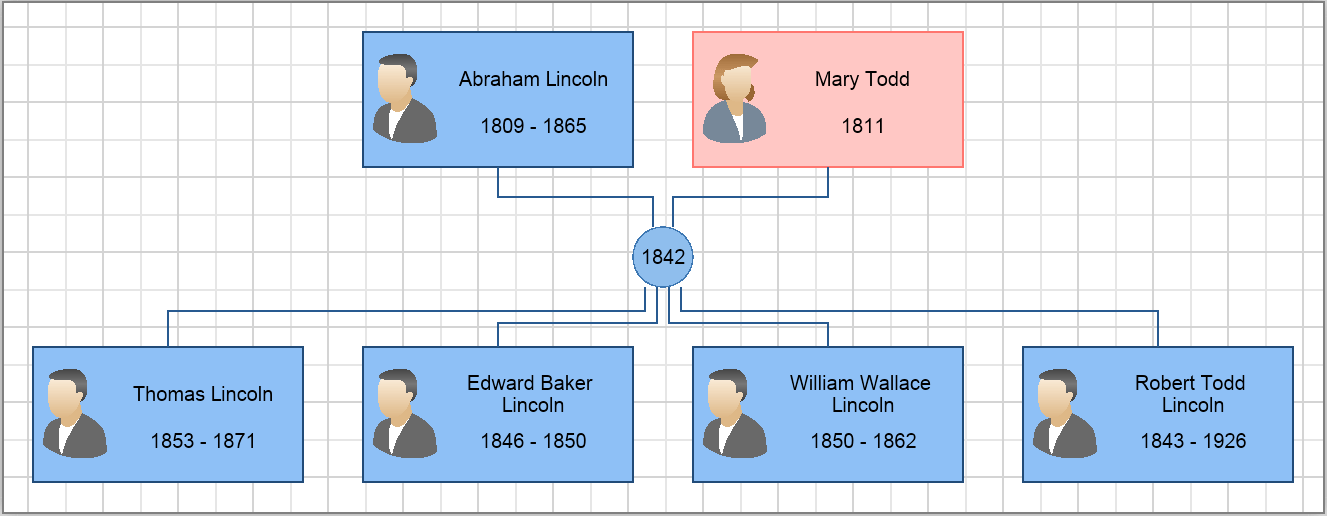
When the user double-clicks a family tree shape, a dialog for editing its properties opens.
To add new family tree shapes interactively right-click a person shape and select Add Parents or Add Spouse, or right-click a relationship shape and select Add Child.
Family Tree Diagram Extension
NOV Diagram comes with diagram extensions, which contain additional information or settings for specific diagram types. Diagram extensions can be specified at drawing level and at page level. The code below demonstrates how to set an NFamilyTreeDiagramExtension to a drawing:
Set family tree diagram extension |
Copy Code
|
drawing.Extensions = new NDiagramExtensionCollection();
drawing.Extensions.Add(new NFamilyTreeExtension());
|
The family tree diagram extension contains the following properties:
- DateFormat - specifies the date format for dates in family tree shapes. By default set to ENDateTimeValueFormat.Year4Digit. Can also be ENDateTimeValueFormat.Date.
- ShowPhotos - determines whether to show person photos or not. Bt default set to true.
When the family tree extension is added to a drawing or a page, a contextual ribbon tab is automatically shown if there's a ribbon associated with the drawing view. That ribbon tab contains a Settings button, which can be used to configure the family tree extension properties.
Arranging Family Trees
To arrange a family tree diagram, use the NFamilyGraphLayout class:
Arranging a family tree |
Copy Code
|
NFamilyGraphLayout layout = new NFamilyGraphLayout();
object[] shapes = page.GetShapes(false).ToArray<object>();
layout.Arrange(shapes, new NDrawingLayoutContext(page));
|
Code Example
The family tree diagram shown on the image above can be created with the following code:
Sample family tree diagram |
Copy Code
|
private void CreateFamilyTree(NPage page)
{
// Create the parents
NShape fatherShape = CreateShape(ENFamilyTreeShape.Male);
fatherShape.SetShapePropertyValue("FirstName", "Abraham");
fatherShape.SetShapePropertyValue("LastName", "Lincoln");
fatherShape.SetShapePropertyValue("BirthDate", new NMaskedDateTime(1809, 02, 12));// NMaskedDateTime
fatherShape.SetShapePropertyValue("DeathDate", new NMaskedDateTime(1865, 04, 15));
page.Items.Add(fatherShape);
NShape motherShape = CreateShape(ENFamilyTreeShape.Female);
motherShape.SetShapePropertyValue("FirstName", "Mary");
motherShape.SetShapePropertyValue("LastName", "Todd");
motherShape.SetShapePropertyValue("BirthDate", new NMaskedDateTime(1811));
page.Items.Add(motherShape);
// Create the children
NShape childShape1 = CreateShape(ENFamilyTreeShape.Male);
childShape1.SetShapePropertyValue("FirstName", "Thomas");
childShape1.SetShapePropertyValue("LastName", "Lincoln");
childShape1.SetShapePropertyValue("BirthDate", new NMaskedDateTime(1853, 4, 4));
childShape1.SetShapePropertyValue("DeathDate", new NMaskedDateTime(1871));
page.Items.Add(childShape1);
NShape childShape2 = CreateShape(ENFamilyTreeShape.Male);
childShape2.SetShapePropertyValue("FirstName", "Robert Todd");
childShape2.SetShapePropertyValue("LastName", "Lincoln");
childShape2.SetShapePropertyValue("BirthDate", new NMaskedDateTime(1843, 8, 1));
childShape2.SetShapePropertyValue("DeathDate", new NMaskedDateTime(1926, 7, 26));
page.Items.Add(childShape2);
NShape childShape3 = CreateShape(ENFamilyTreeShape.Male);
childShape3.SetShapePropertyValue("FirstName", "William Wallace");
childShape3.SetShapePropertyValue("LastName", "Lincoln");
childShape3.SetShapePropertyValue("BirthDate", new NMaskedDateTime(1850, 12, 21));
childShape3.SetShapePropertyValue("DeathDate", new NMaskedDateTime(1862, 2, 20));
page.Items.Add(childShape3);
NShape childShape4 = CreateShape(ENFamilyTreeShape.Male);
childShape4.SetShapePropertyValue("FirstName", "Edward Baker");
childShape4.SetShapePropertyValue("LastName", "Lincoln");
childShape4.SetShapePropertyValue("BirthDate", new NMaskedDateTime(1846, 3, 10));
childShape4.SetShapePropertyValue("DeathDate", new NMaskedDateTime(1850, 2, 1));
page.Items.Add(childShape4);
// Create the relationship shape
NShape relShape = CreateShape(ENFamilyTreeShape.Relationship);
relShape.SetShapePropertyValue("MarriageDate", new NMaskedDateTime(1842, 11, 4));
page.Items.Add(relShape);
page.Items.Add(CreateConnector(fatherShape, relShape));
page.Items.Add(CreateConnector(motherShape, relShape));
page.Items.Add(CreateConnector(relShape, childShape1));
page.Items.Add(CreateConnector(relShape, childShape2));
page.Items.Add(CreateConnector(relShape, childShape3));
page.Items.Add(CreateConnector(relShape, childShape4));
// Arrange the family tree shapes
NFamilyGraphLayout layout = new NFamilyGraphLayout();
object[] shapes = page.GetShapes(false).ToArray<object>();
layout.Arrange(shapes, new NDrawingLayoutContext(page));
// Size the page to its content
page.SizeToContent();
}
|