Integrating NOV in Mac with JetBrains Rider
In This Topic
Nevron Open Vision (NOV) can be integrated in Mac application projects. This topic provides a step-by-step tutorial on how to achieve this. This guide is for the JetBrains Rider IDE.
Download and Install NOV
Download the NevronOpenVision.Mac package.
After you install the package go to Applications and launch Nevron Open Vision. The application has the following UI items:
- Start NOV Examples App - when pressed it will launch the NOV examples for Mac.
- Open NOV Examples Project - when pressed it will launch "Finder" and open the NOV Examples folder. You can double click on the .sln file contained there ("Nevron.Nov.Examples.XamarinMac.sln") in order to open the examples in JetBrains Rider.
- Open NOV Binary Files - when pressed it will launch "Finder" and open the NOV Bin folder, which contains the redistributable dlls of NOV for Xamarin Mac.
- View NOV Documentation - when pressed it will launch your default browser and open the NOV online documentation.
Below those buttons you can also view the generated machine id and the current evaluation key.
- Machine Id - contains the current machine id. This machine id is used to generate a development license key - see Activation for more details.
- License Key(s) - contains the license key currently applied on the examples. At install time the NOV installation will automatically generate a 60 days trial evaluation key which you can also use in your projects for testing.
Integrate in a Mac Application Project
The instructions below show how to integrate Nevron Open Vision (NOV) in a Mac Application project created with JetBrains Rider for Mac. If you want to download a ready project, which you can directly compile and run with JetBrains Rider for Mac, click here to download the NovMacApp project. All you have to do is to extract the project to a directory of your choice on your Mac and add the correct references to the NOV assemblies. Then go directly to step 7 - "Run the application".
If you prefer to follow the instructions instead of loading the ready project or you want to integrate NOV in an existing Mac Application project, then you can use the following instructions.
In this step by step tutorial, we are going to create a very simple Mac application. In such applications, the entire UI is created from code only and we'll have to create a window and a window controller class. Fortunately, this is very simple and takes a minimal amount of code.
-
Run JetBrains Rider on your Mac and create a new Console project
- Click the "New Solution" button at the top.
- Select the "Console" project type.
- Enter the name of your Mac application, for example "NovMacApp".
- Select target framework, for example "net8.0".
- Check the "Put project and solution in the same directory" and the "Do not use top-level statements" options.
This step is not mandatory, because you can integrate NOV in an already existing Mac Application project. It is performed just for the purpose of making a complete installation scenario.
-
Change the project type of a macOS application
- Right-click the project and select Edit -> Edit "NovMacApp.csproj"
- Add a "-macos" suffix to the target framework of the project, for example "<TargetFramework>net8.0-macos</TargetFramework>"
-
Reference the NOV Assemblies
Right-click Dependencies in the solution explorer, select Reference, click the Add From button and add the following NOV DLLs as references to your project:
- Nevron.Nov.Presentation.dll - core NOV assembly. Contains common NOV UI widgets like labels, buttons, drop downs, menus, toolbars, ribbon, etc.
- Nevron.Nov.Host.Mac.dll - NOV presentation host for macOS.
- Nevron.Nov.Barcode - barcode component.
- Nevron.Nov.Chart - chart component.
- Nevron.Nov.Diagram - diagram component. Requires the Barcode, Text and Grid components.
- Nevron.Nov.Grid - grid component.
- Nevron.Nov.Schedule - schedule component. Requires the Barcode component.
- Nevron.Nov.Text - rich text component. Requires the Barcode component.
- Nevron.Nov.RoslynCompilerService - add this reference if you plan to use diagram shapes with code-behind like family tree shapes.
You can reference the dlls directly from the System/Applications/NevronOpenVision.Mac/Contents/Bin folder or copy those dlls to a folder which is more convenient for referencing.
-
Place the following code in the "Program.cs" file of the project
Install NOV |
Copy Code
|
using Nevron.Nov;
using Nevron.Nov.Mac;
using Nevron.Nov.Text;
using Nevron.Nov.Chart;
using Nevron.Nov.Diagram;
using Nevron.Nov.Schedule;
using Nevron.Nov.Grid;
using Nevron.Nov.Barcode;
using Nevron.Nov.Compiler;
namespace NovMacApp
{
class Program
{
static void Main(string[] args)
{
NSApplication.Init();
// Apply license for redistribution here. You can skip this code when evaluating NOV.
// NLicenseManager.Instance.SetLicense(new NLicense("LICENSE KEY"));
// Install the NOV Mac integration services
NNovApplicationInstaller.Install<MainWindow>(
NBarcodeModule.Instance,
NTextModule.Instance,
NGridModule.Instance,
NChartModule.Instance,
NDiagramModule.Instance,
NScheduleModule.Instance);
// If you plan to use diagram shapes with code-behind like family tree shapes,
// set the compiler service
NApplication.CompilerService = new NRoslynCompilerService();
NSApplication.SharedApplication.Run();
}
}
}
|
-
Add a MainWindow.cs file
Right click on the NevronTestApp project and select Add New File / Empty Class. The name of the class should be "MainWindow" and it should have a parameterless constructor:
Installing NOV for Xamarin.Mac |
Copy Code
|
using Nevron.Nov.Mac;
using Nevron.Nov.UI;
namespace NovMacApp
{
public partial class MainWindow : NSWindow
{
#region Constructors
public MainWindow()
: base(new CGRect(0, 0, 400, 400), NSWindowStyle.Resizable | NSWindowStyle.Titled | NSWindowStyle.Closable, NSBackingStore.Buffered, false)
{
Initialize();
}
#endregion
#region Implementation
/// <summary>
/// Shared initialization code.
/// </summary>
private void Initialize()
{
Title = "My NOV Application";
SetFrame(NSScreen.MainScreen.VisibleFrame, true);
// place the host inside the mac window
ContentView = new NNovWidgetHost(CreateNovContent());
}
private NWidget CreateNovContent()
{
return new NLabel("Hello from Nevron Open Vision!");
}
#endregion
}
}
|
This code creates a new NOV widget host that contains a simple label. The content of the NOV widget host should be set to a NOV widget and can be any NOV widget, for example a label, a button, a document view (drawing view, schedule view, rich text view, etc.) a panel with other widgets and so on. See the UI Overview topic for an overview of the NOV User Interface.
-
Add an "Info.plist" file and a bundle identifier for the application
- Right-click the project and select "Add -> Property List". Name it "Info.plist".
- Click the plus button and add "Bundle Identifier".
- Set a value for the bundle identifier, for example "com.nov.app".
- Run the Application
Select the NovMacApp with the mini Apple logo and run the application:
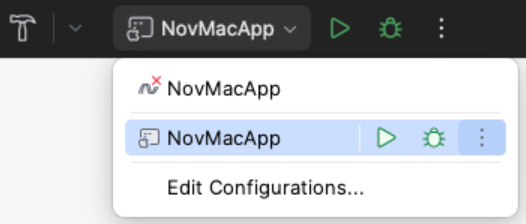
Congratulations you've just created your first Mac app with Nevron Open Vision.
See Also