In This Topic
The NOV Flex Box panel is a directed layout panel that arranges its child widgets based on the values of their Grow and Shrink extended properties just like the CSS Flexbox does. It makes it easy to configure how widgets should occupy the available area of the panel. Consider it as a more flexible and powerful version of the Stack Panel.
The Grow and Shrink Extended Properties
The Grow and Shrink of all widgets are by default 0. Negative values are not allowed.
There are 2 ways to set the Grow and Shrink extended properties:
- Implicitly by passing the values to the Add or Insert method of a flex box panel. The first parameter specifies the widget to add, the second - the Grow property value and the third - the Shrink property value. If you pass Double.NaN for grow or shrink, the respective extended property will not be set. The following line of code demonstrates how to add a widget to a flex box panel and set it's Grow and Shrink extended properties implicitly:
Setting Grow and Shrink implicitly |
Copy Code
|
flexBoxPanel.Add(widget, 1, 1);
|
- Explicitly by using the SetGrow and SetShrink static methods of the NFlexBoxLayout class, for example:
Setting Grow and Shrink explicitly |
Copy Code
|
NFlexBoxLayout.SetGrow(widget, 1);
NFlexBoxLayout.SetShrink(widget, 1);
flexBoxPanel.Add(widget);
|
The NFlexBoxLayout class also provides the following static methods:
- GetGrow, GetShrink - get the Grow and Shrink extended properties of a widget.
- ClearGrow, ClearShrink - clear the Grow and Shrink extended properties of a widget.
Code Example
Let's take a look at the following example - we have 3 labels added to a flex box panel, where:
- The first label has Grow and Shrink set to 1
- The second label has Grow and Shrink set to 3
- The third label does not have Grow and Shrink values set
In this case the first label will occupy 1 / (1 + 3) = 1 / 4 (i.e. 25%) of the available area and the second label will occupy 3 / (1 + 3) = 3 / 4 (i.e. 75%) of the available area as shown on the following image:
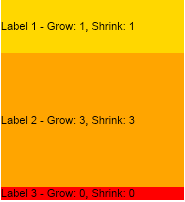
To create the flex box panel above, use the following code:
Using a Flex Box Panel |
Copy Code
|
NFlexBoxPanel panel = new NFlexBoxPanel();
panel.PreferredHeight = 200;
// Set the Grow and Shrink extended properties of the first label explicitly
NLabel label1 = new NLabel("Label 1 - Grow: 1, Shrink: 1");
label1.BackgroundFill = new NColorFill(NColor.Gold);
NFlexBoxLayout.SetGrow(label1, 1);
NFlexBoxLayout.SetShrink(label1, 1);
panel.Add(label1);
// Pass the values of the Grow and Shrink extended properties of the second label
// to the Add method of the panel
NLabel label2 = new NLabel("Label 2 - Grow: 3, Shrink: 3");
label2.BackgroundFill = new NColorFill(NColor.Orange);
panel.Add(label2, 3, 3);
// The third label will have the default values for Grow and Shrink - 0
NLabel label3 = new NLabel("Label 3 - Grow: 0, Shrink: 0");
label3.BackgroundFill = new NColorFill(NColor.Red);
panel.Add(label3);
|
See Also