Column Conditional Formatting
In This Topic
About Conditional Formatting
Conditional formatting helps you format certain data cell views, according to specific rules. The conditional formatting capabilities of a column format, are exposed as a collection of NFormattingRule instances that are contained inside a NFormattingRuleCollection accessible from the NColumnFormat.FormattingRules property.
Each formatting rule is a couple that packs a Row Condition together with a collection formatting declarations. The formatting rules of a column are represented by instances of the NFormattingRule class and are contained inside a NFormattingRuleCollection, accessible from the FormattingRules property of the NColumn class.
The formatting declarations are applied to a certain data cell view, if the row condition matches the data cell row value. The following code example applies a red background to all data cell views for which the sales value is larger than 500:
Conditional Formatting Example |
Copy Code
|
// create a dummy data table
NMemoryDataTable dataTable = new NMemoryDataTable(
new NFieldInfo("Company", typeof(string)),
new NFieldInfo("Sales", typeof(double))
);
dataTable.AddRow("Egestas Duis Ltd", 347);
dataTable.AddRow("Vel Turpis Aliquam LLC", 852);
dataTable.AddRow("Ullamcorper Velit Associates", 724);
// create grid view and get its grid
NTableGridView gridView = new NTableGridView();
NTableGrid grid = gridView.Grid;
// bind the grid to data
grid.DataSource = new NDataSource(dataTable);
// get the sales column and apply conditional formatting to it
NColumn salesColumn = grid.Columns.GetColumnByFieldName("Sales");
// create a new formatting rule and add it the rules collection
NFormattingRule formattingRule = new NFormattingRule();
salesColumn.FormattingRules.Add(formattingRule);
// create a row condition that matches Sales Greater than 500
formattingRule.RowCondition = new NOperatorRowCondition(new NFieldRowValue("Sales"), ENRowConditionOperator.GreaterThan, "500");
// create a red background fill declaration
formattingRule.Declarations.Add(new NBackgroundFillDeclaration(new NColorFill(NColor.Red)));
|
Formatting Declarations Hierarchy
The formatting declarations are represented by classes that derive from the NFormattingDeclaration class. The following image illustrates the current formatting declarations hierarchy:
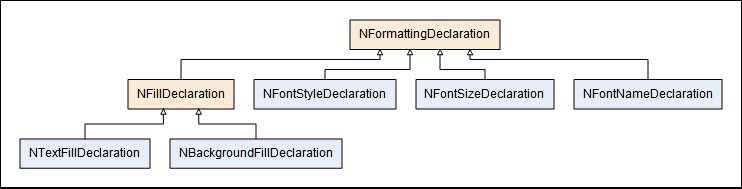
Currently there are two general types of formatting declarations - fill declarations (for text and background) and font style declarations (for font name, size and style).
Fill Formatting Declarations
The fill formatting declarations derive from the base NFillDeclaration class. Since all data cell views derive from the base NWidget class, the NTextFillDeclaration and NBackgroundFillDeclaration can effectively be applied to all types of columns.
The respective fillings applied (to the background of the data cell view widget or to the font fill) is determined by the Mode property, which takes values from the ENFillDeclarationMode enumeration:
-
Uniform - in this mode the filling of all data cell view widgets is the same, and is determined by the UniformFill. The following code example creates a NTextFillDeclaration that applies an uniform red fill to the text of all column cells:
Uniform Fill |
Copy Code
|
NTextFillDeclaration textFillDeclaration = new NTextFillDeclaration();
textFillDeclaration.Mode = ENFillDeclarationMode.Uniform;
textFillDeclaration.UniformFill = new NColorFill(NColor.Red);
|
-
TwoColorGradient - in this mode the filling of each data cell view is determined by the respective column value, that defines an interpolated color based on a color ramp defined by the BeginColor and EndColor properties. The following example applies a two color gradient background fill to the sales column:
Gradient Fill |
Copy Code
|
// create a background fill declaration
NBackgroundFillDeclaration backgroundFillDeclaration = new NBackgroundFillDeclaration();
// the color filling ranges from red to green
backgroundFillDeclaration.Mode = ENFillDeclarationMode.TwoColorGradient;
backgroundFillDeclaration.BeginColor = NColor.Red;
backgroundFillDeclaration.EndColor = NColor.Green;
// the color ramp minimum and maximum are extracted from the data source.
object min, max;
grid.DataSource.TryGetMin("Sales", out min);
grid.DataSource.TryGetMax("Sales", out max);
backgroundFillDeclaration.MinimumValue = Convert.ToDouble(min);
backgroundFillDeclaration.MaximumValue = Convert.ToDouble(max);
formattingRule.Declarations.Add(backgroundFillDeclaration);
|
- ThreeColorGradient - the same as TwoColorGradient except that the color ramp contains an additional middle color, defined by the MiddleColor property.
Font Formatting Declarations
There are three types font formatting declarations, that can affect the text formatting of NTextCellView data cell view widgets:
- NFontNameDeclaration - applies a font name.
- NFontSizeDeclaration - applies a font size.
- NFontStyleDeclaration - applies a font style (e.g. bold, italic, underline).
Note that the formatting declarations will only have effect if applied to a column, whose column format generates
NTextCellView widgets. See the
Formats Table for an overview of the column formats that generate
NTextCellView widgets.
See Also