In This Topic
About Filtering
Filtering is the process of passing only certain records from the data source to be displayed by the grid. The filtering is the first data processing step performed by both the Table Grid and the Tree Grid. The filtering of a grid is defined by a collection of NFilteringRule instances that are contained inside the NFilteringRuleCollection collection accessible from the NGrid.FilterRules property.
Each NFilteringRule defines a predicate (i.e. a function that evaluates to true or false). In order for a record from the data source to be displayed by the grid, the record must pass all filtering rules - i.e. for that record all filter predicates must return true. The filtering rule predicate is specified by a Row Condition.
Each NFilteringRule can be optionally associated with a grid column. This is achieved by setting the NFilteringRule.Column property to reference the column that you want to associate with the rule. When a filter rule is associated with a column, by default that column serves as a row value provider for the row condition that the filtering rule aggregates.
The following code example creates two filtering rules:
Filter Rules Example |
Copy Code
|
// create a view and get its grid
NTableGridView tableView = new NTableGridView();
NTableGrid tableGrid = tableView.Grid;
// bind to data source
NMemoryDataTable dataTable = new NMemoryDataTable(new NFieldInfo("Price", typeof(double)), new NFieldInfo("Quantity", typeof(int)));
dataTable.AddRow(20.0d, 4);
dataTable.AddRow(30.0d, 5);
dataTable.AddRow(40.0d, 5);
tableGrid.DataSource = new NDataSource(dataTable);
// create a filter rule that only passes records for which the value of the Price is greater than 25
tableGrid.FilteringRules.Add(new NFilteringRule(tableGrid.Columns.GetColumnByFieldName("Price"), ENRowConditionOperator.GreaterThan, "25"));
// create a filter rule that only passes records for which the value of the Price is less than 25
tableGrid.FilteringRules.Add(new NFilteringRule(tableGrid.Columns.GetColumnByFieldName("Price"), ENRowConditionOperator.LessThan, "35"));
|
Filtering Rule and its Row Condition
Because the NFilteringRule represent a row predicates, there is a row condition associated with each filter rule, which is specified by the RowCondition property of the filtering rule. If the filtering rule does not have a valid row condition defined, the filtering rule is ignored (not taken into account). Most of the constructors of NFilteringRule allow you to indirectly specify a row condition. You can also specify the row condition via the RowCondition property:
Filtering Rule Row Condition |
Copy Code
|
NFilteringRule filteringRule = new NFilteringRule();
NOperatorRowCondition rowCondtion = new NOperatorRowCondition();
rowCondtion.RowValue = new NFieldRowValue("Sales");
rowCondtion.Operator = ENRowConditionOperator.GreaterThan;
rowCondtion.Value = "100";
filteringRule.RowCondition = rowCondtion;
|
Filtering Rule and the associated Column
Each filtering rule can be associated with a grid column. The associated column is specified by the NFilteringRule.Column property. There are several things, which happen when a filtering rule is associated with a column:
- Filtering Indicator - When there is at least one filtering rule that is associated with a specific column, the column header will visually indicate that the grid is filtered by that column as illustrated on the following image:
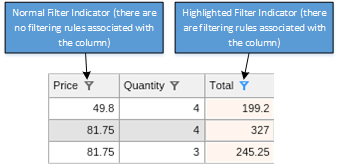
- Filtering Dialog - the filtering rule will appear in the list of filtering rules associated with the specific column. The filtering dialog is invoked when the user clicks on the filtering indicator:
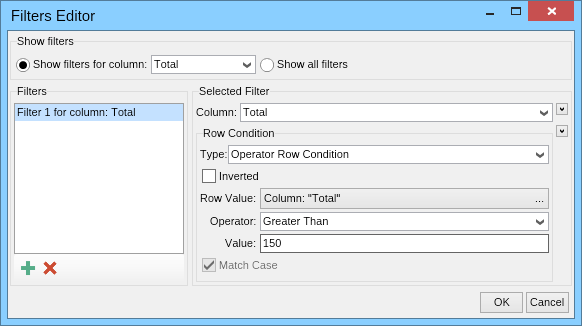
-
Row Value Provider - the filtering rule by default serves as a row value provider, by providing row values from the associated column. If a row value is not specified explicitly in the associated row condition, the associated column serves as row value provider. The following code example creates a filter rule with an operator row condition, which does not have an explicit row value:
Column serving as Row Value Provider |
Copy Code
|
// create a filtering rule that filters totalColumn values which are greater than 100.
// internally the constructor creates an NOperatorRowCondition which does not have an associated row value,
// however since the filtering rule is associated with the totalColumn,
// the condition uses the totalColumn as a row value provider.
NFilteringRule filteringRule = new NFilteringRule(totalColumn, ENRowConditionOperator.GreaterThan, "100");
|
See Also