Block elements derive directly or indirectly from the NBlock base class.
The following class hierarchy shows the different block elements that can reside in the text document:
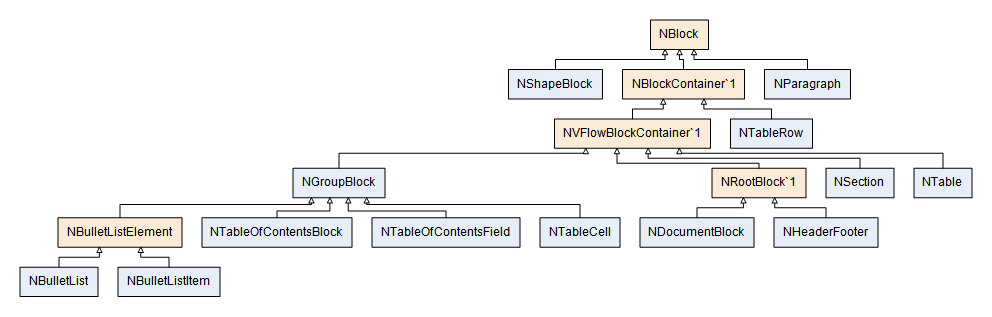
From this diagram you can see that only three classes derive directly from NBlock – NShapeBlock, NParagraph, and NBlockContainer. The NShapeBlock and NParagraphBlock contain inlines only, whereas the NBlockContainer descendants can contain other blocks. Some container blocks require specific child blocks - for example NTableRow can contain only NTableCell child blocks. In general, there are three types of content blocks:
- Root Blocks - The blocks in this category are represented by the NDocumentBlock and NHeaderFooter blocks. They represent blocks that form a root for text position and selection.
- Content Blocks - The blocks in this category are the NParagraph, NShapeBlock, NGroupBlock, and NTable. These blocks can be present as child blocks in the root block, table cells, and group blocks. The NParagraph and NShapeBlock blocks cannot contain child blocks - they can contain only inline elements.
- Intrinsic Parent Blocks - The blocks in this category can appear in the document tree only as child blocks of other blocks. The blocks in this category are the NSection (only as a child of a document block), NTableRow (only as a child of a NTable block), and NTableCell (only as a child of NTableRow).
The following containment diagrams show how blocks can be nested in each other:
NDocumentBlock and NSection
The document block is the root block in the document tree. It can contain only section blocks as illustrated by the following diagram:
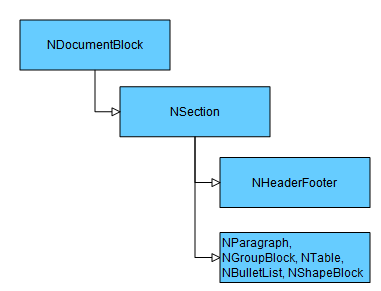
In turn each section in the document can have header/footer blocks and other content – paragraphs, tables, shapes, or group blocks.
The following code snippet shows how to add two sections with different page sizes to a document:
Adding Multiple Sections |
Copy Code
|
NSection section1 = new NSection();
NParagraph paragraph1 = new NParagraph("Paragraph1");
section1.Blocks.Add(paragraph1);
section1.PaperSize = new NPaperSize(ENPaperKind.A3);
m_RichText.Content.Sections.Add(section1);
NSection section2 = new NSection();
NParagraph paragraph2 = new NParagraph("Paragraph2");
section2.Blocks.Add(paragraph2);
section2.PaperSize = new NPaperSize(ENPaperKind.A4);
m_RichText.Content.Sections.Add(section2);
|
Header and footer elements are represented by instances of the NHeaderFooter class. Only sections can contain header / footer elements, which are visible when the control operates in Print layout mode. In turn header/footer elements can contain any number of NParagraph, NGroupBlock, NTable, and NShapeBlock blocks:
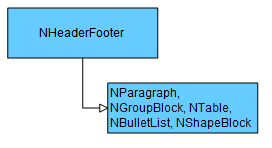
The following code snippet shows how to create a section with a header:
Adding Section Header |
Copy Code
|
NSection section = new NSection();
// create the header
NHeaderFooter header = new NHeaderFooter();
// create a paragraph containing a page number field
NParagraph headerParagraph = new NParagraph();
headerParagraph.Inlines.Add(new NFieldInline(ENNumericFieldName.PageNumber));
header.Blocks.Add(headerParagraph);
section.DifferentFirstHeaderAndFooter = false;
section.DifferentOddEvenHeadersAndFooters = false;
section.Header = header;
// create some section content
section.Blocks.Add(new NParagraph("Section Text"));
m_RichText.Content.Sections.Add(section);
|
The group block element is used to group one or more content blocks. The following containment diagram shows this:
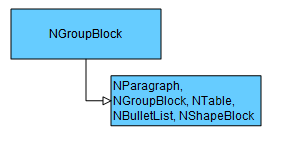
The following snippet shows how to create two group blocks each containing several paragraphs. The second group will be displayed on the next page if the control uses a paging view:
Adding Group Blocks |
Copy Code
|
NSection section = new NSection();
// create a group block
NGroupBlock group1 = new NGroupBlock();
group1.BackgroundFill = new NStockGradientFill(NColor.LightBlue, NColor.LightGray);
group1.Blocks.Add(new NParagraph("Paragraph 1"));
group1.Blocks.Add(new NParagraph("Paragraph 2"));
group1.Blocks.Add(new NParagraph("Paragraph 3"));
section.Blocks.Add(group1);
// create a group block
NGroupBlock group2 = new NGroupBlock();
group2.PageBreakBefore = true;
group2.BackgroundFill = new NStockGradientFill(NColor.LightBlue, NColor.LightGray);
group2.Blocks.Add(new NParagraph("Paragraph 1"));
group2.Blocks.Add(new NParagraph("Paragraph 2"));
group2.Blocks.Add(new NParagraph("Paragraph 3"));
section.Blocks.Add(group2);
m_RichText.Content.Sections.Add(section);
|
The table block is represented by the following containment diagram:
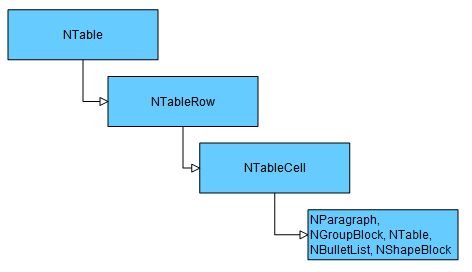
Each table consists of rows (NTableRow) and each row consists of table cells. In turn table cells can contain paragraphs, group blocks, bullet lists, or other tables.
The table model in Nevron Rich Text is described later in detail. For now, let's take a look at a simple example that creates a 2x2 table:
Adding a Table |
Copy Code
|
NSection section = new NSection();
// create a table
NTable table = new NTable();
table.BackgroundFill = new NStockGradientFill(NColor.LightBlue, NColor.LightGray);
// add two columns
table.Columns.Add(new NTableColumn());
table.Columns.Add(new NTableColumn());
// add two rows
NTableRow tableRow1 = new NTableRow();
// add two cells
tableRow1.Cells.Add(new NTableCell(new NParagraph("Cell 11")));
tableRow1.Cells.Add(new NTableCell(new NParagraph("Cell 12")));
table.Rows.Add(tableRow1);
NTableRow tableRow2 = new NTableRow();
// add two cells
tableRow2.Cells.Add(new NTableCell(new NParagraph("Cell 21")));
tableRow2.Cells.Add(new NTableCell(new NParagraph("Cell 22")));
table.Rows.Add(tableRow2);
section.Blocks.Add(table);
|