Inline elements generally appear in paragraphs. They represent either content that must be displayed on a line or are used to alter the formatting of that line. For example, image and text inlines represent content, whereas a line break or page break inline element represents a formatting instruction.
In addition, there are several inline elements that are called intrinsic inline elements and they are associated with their containing block – these are the paragraph end, bullet inline and section break inline. You cannot add or delete intrinsic inline elements – they will appear in the document when you add a block element that contains an intrinsic inline – for example when you add a paragraph to the document tree this automatically implies that you add the paragraph end inline at the end of the paragraph block.
Now let's take a look at how to programmatically add a few text inlines to a paragraph with different font name, font size and font style:
Adding Inline Elements to Paragraphs |
Copy Code
|
NSection section = new NSection();
m_RichText.Content.Sections.Add(section);
NParagraph paragraph = new NParagraph();
NTextInline textInline2 = new NTextInline("Font Name (Tahoma), ");
textInline2.FontName = "Tahoma";
paragraph.Inlines.Add(textInline2);
NTextInline textInline3 = new NTextInline("Font Size (14pt), ");
textInline3.FontSize = 14;
paragraph.Inlines.Add(textInline3);
NTextInline textInline4 = new NTextInline("Font Style (Bold).");
textInline4.FontStyle = ENFontStyle.Bold;
paragraph.Inlines.Add(textInline4);
section.Blocks.Add(paragraph);
|
This code produces the following output:

All inline elements regard a list of properties that can be either specified locally or from a style sheet. Some properties can also be inherited from the inline parent block.
Font Properties
FontName
Specifies the font name – for example “Arial”, “Tahoma”, “Times New Roman”. The following code snippet shows how to modify the font name:
Font Name |
Copy Code
|
someInline.FontName = "Times New Roman";
|
FontStyle
Specifies the font style as a bitmask of the values contained in the
ENFontStyle enumeration:
Font Size |
Copy Code
|
someInline.FontSize = 16; // make the font 16pt
|
ENFontStyle |
Description |
Regular |
The font style is regular. |
Bold |
The font style is bold. |
Italic |
The font style is italic. |
Underline |
The text is displayed with an underline. |
Strikethrough |
The text is displayed with a strikethrough. |
Since the values of the ENFontStyle are bit values you can specify combinations – the following code snippet for example specifies a bold/italic font:
Font Style |
Copy Code
|
textInline.FontStyle = ENFontStyle.Bold | ENFontStyle.Italic;
|
Baseline
The baseline property controls whether the displayed text must have normal, superscript, or subscript baseline. The baseline also affects the effective font size in case you specify superscript or subscript depending on the currently used font preferences. The following table lists the available options:
ENBaseLine |
Description |
Normal |
The text is displayed normally. |
Superscript |
The text is displayed as superscript. Superscript text appears at an elevated baseline position and smaller font size – for example, x2. |
Subscript |
The text is displayed as a subscript. Superscript text appears at a lower baseline position and smaller font size – for example x2. |
The following example shows how to display “E=MC2”:
Baseline |
Copy Code
|
NSection section = new NSection();
m_RichText.Content.Sections.Add(section);
NParagraph paragraph = new NParagraph();
NTextInline text1 = new NTextInline("E=MC");
paragraph.Inlines.Add(text1);
NTextInline text2= new NTextInline("2");
text2.Baseline = ENBaseLine.Superscript;
paragraph.Inlines.Add(text2);
section.Blocks.Add(paragraph);
|
Panose
The Panose number is a ten-byte number that describes basic font characteristics, such as whether the font uses serifs, the thickness of the glyphs, etc. Each TrueType font has a Panose number, which can be used to select an alternative font if the specified font is not available on the system. The control will choose the closest matching font from the installed fonts based on the smallest distance from the specified Panose number. The following code snippet, for example, specifies the Panose number for "Arial" and sets the font name to a non-existing font:
Panose |
Copy Code
|
NTextInline text1 = new NTextInline("Arial");
text1.FontName = "UnknownFont";
text1.Panose = new NPanoseNumber(new byte[] { 2, 11, 6, 4, 2, 2, 2, 2, 2, 4 });
paragraph.Inlines.Add(text1);
|
The result is that the text will be displayed with “Arial” as the specified Panose number most closely matches that font.
Font Character and Word Spacing
The FontCharacterSpacing and FontWordSpacing properties allow you to apply additional spacing between characters and words in the displayed text. The FontCharacterSpacing property applies spacing in dips between every two adjacent characters in the text, whereas the FontWordSpacing applies additional spacing after each space character, thus increasing the distance between words in the text document. Both properties accept negative values so you can also use them to condense or to expand the displayed text.
Panose |
Copy Code
|
NTextInline text1 = new NTextInline("This text has applied additional character and word spacing");
text1.FontCharacterSpacing = 1; // increase the distance between characters with 1 DIP
text1.FontWordSpacing = 2; // increase the distance between words with 2 DIPs
paragraph.Inlines.Add(text1);
|
The result is that the text will be displayed with increased character and word spacing.
Appearance Properties
Fill
The fill property specifies the filling applied to text – the following code snippet displays a text with gradient filling:
Fill |
Copy Code
|
NSection section = new NSection();
m_RichText.Content.Sections.Add(section);
NParagraph paragraph = new NParagraph();
NTextInline text1 = new NTextInline("Text with Gradient Filling");
text1.Fill = new NStockGradientFill(NColor.Red, NColor.Orange);
text1.FontSize = 16;
paragraph.Inlines.Add(text1);
section.Blocks.Add(paragraph);
|
This code results in the following text output:

BackgroundFill / HighlightFill
The background and highlight fill allow you to apply background filling on text. In case both properties are specified the highlight takes precedence. The following code snippet shows how to display text consisting of two inlines with different background filling:
Background Fill / Highlight Fill |
Copy Code
|
NSection section = new NSection();
m_RichText.Content.Sections.Add(section);
NParagraph paragraph = new NParagraph();
NTextInline text1 = new NTextInline("Text with Black Background");
text1.Fill = new NColorFill(NColor.White);
text1.BackgroundFill = new NColorFill(NColor.Black);
text1.FontSize = 16;
paragraph.Inlines.Add(text1);
|
This code results in the following text output:

Stroke
One of the unique features of Nevron Text is that it allows you to apply stroke on text. The following code snippet shows how to create artistic text:
Artistic Text |
Copy Code
|
NSection section = new NSection();
m_RichText.Content.Sections.Add(section);
NParagraph paragraph = new NParagraph();
NTextInline text1 = new NTextInline("This is Artistic Text");
text1.Fill = new NStockGradientFill(ENGradientStyle.Horizontal, ENGradientVariant.Variant1, NColor.White, NColor.Red);
text1.Stroke = new NStroke(1, NColor.Yellow);
text1.FontSize = 22;
paragraph.Inlines.Add(text1);
|
This code results in the following text output:
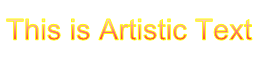