Paragraph blocks consist of inline elements, which are laid out horizontally line by line. Some inline elements represent content while others represent a formatting instruction. This topic discusses the different paragraph formatting options.
The paragraph alignment is specified from the HorizontalAlignment property of the paragraph. It accepts values from the ENAlign enum and the options are listed in the following table:
Value |
Description |
Left |
Paragraph content is left aligned |
Center |
Paragraph content is center aligned |
Right |
Paragraph content is right aligned |
Justify |
Paragraph content is justified. Justification will increase the spacing between words so that begin and end words on each line lie on the paragraph content bounds |
The following code snippet shows how to change the horizontal alignment of a paragraph:
Changing Paragraph Horizontal Alignment |
Copy Code
|
NParagraph paragraph = new NParagraph("This is center aligned paragraph");
paragraph.HorizontalAlignment = ENAlign.Center;
section.Blocks.Add(paragraph);
|
First Line Indent and Hanging Indent
The paragraph block supports two properties called FirstLineIndent and HangingIndent that allow you to modify the initial position of the first line of text and subsequent lines of text respectively. By default, both properties are set to zero dips. The following code snippet shows how to create a paragraph that has a negative first line indent and a positive hanging indent:
Changing Paragraph Horizontal Alignment |
Copy Code
|
for (int i = 0; i < 10; i++)
{
s += "Paragraph with modified first line indent and hanging indent. ";
}
NParagraph paragraph = new NParagraph(s);
paragraph.HangingIndent = 10;
paragraph.FirstLineIndent = -10;
paragraph.HorizontalAlignment = ENAlign.Justify;
section.Blocks.Add(paragraph);
|
This code produces the following output:
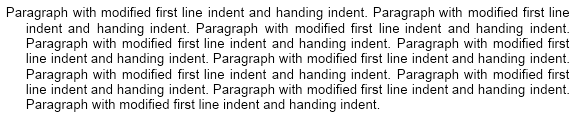
Each line in a paragraph is characterized by three metrics – Ascent, Descent, and Line Gap. They are automatically computed based on the fonts and their sizes that appear on this line. The following picture shows how these three line parameters work together to determine the offset to the next line position:
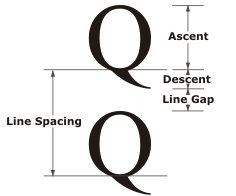
In some cases, you may want to increase or decrease the spacing between lines in a paragraph. This is achieved from the LineHeightMode property accepting values from the ENLineHeightMode enum. The following table lists the available options:
ENLineHeightMode |
Description |
AtLeast |
The line height is computed as the maximum value between the automatic line height and the LineHeight property of the paragraph |
Exactly |
The line height is exactly the LineHeight property of the paragraph |
Multiple |
The line height is computed as a multiple of the automatic line height. The multiplication factor is controlled by the LineHeightFactor property of the paragraph |
The following code snippet shows how to increase the line height:
Changing Line Height |
Copy Code
|
paragraph.LineHeightMode = ENLineHeightMode.Multiple;
paragraph.LineHeightFactor = 2.0;
|
The tab stop concept originates from typewriters, where pressing the tab key moves the carriage to a predefined position. Text processors extend this idea by allowing tab stops to adjust the flow of text relative to these predefined positions.
A tab stop is a preference where the current line character position should be moved when it encounters a NTabInline object. It is specified by adding objects of type NTabStop to the TabStops collection of the paragraph. If you do not specify tab stops then the control will automatically create tab stops based on the DefaultTabWidth property of the NDocumentBlock element.
The following example shows how to create a paragraph with tab stops and tab inlines programmatically:
Tab Stops |
Copy Code
|
NParagraph paragraph = new NParagraph();
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Left."));
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Right."));
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Center."));
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Decimal 345.33"));
NTabStopCollection tabStops = new NTabStopCollection();
tabStops.Add(new NTabStop(100, ENTabStopAlignment.Left, ENTabStopLeaderStyle.None));
tabStops.Add(new NTabStop(200, ENTabStopAlignment.Right, ENTabStopLeaderStyle.None));
tabStops.Add(new NTabStop(300, ENTabStopAlignment.Center, ENTabStopLeaderStyle.None));
tabStops.Add(new NTabStop(500, ENTabStopAlignment.Decimal, ENTabStopLeaderStyle.None));
paragraph.TabStops = tabStops;
section.Blocks.Add(paragraph);
|
The resulting image of this paragraph is illustrated by the following picture:

In this picture, the red lines show the tab stop positions. You can see that the tab stop alignment will alter the normal flow of text in the paragraph.
You can also alter the tab leader style. This property allows you to insert a repeating char or line at the space occupied by the tab stop:
Changing the Tab Leader Style |
Copy Code
|
NParagraph paragraph = new NParagraph();
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Left."));
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Right."));
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Center."));
paragraph.Inlines.Add(new NTabInline());
paragraph.Inlines.Add(new NTextInline("Decimal 345.33"));
NTabStopCollection tabStops = new NTabStopCollection();
tabStops.Add(new NTabStop(100, ENTabStopAlignment.Left, ENTabStopLeaderStyle.Dots));
tabStops.Add(new NTabStop(200, ENTabStopAlignment.Right, ENTabStopLeaderStyle.EqualSigns));
tabStops.Add(new NTabStop(300, ENTabStopAlignment.Center, ENTabStopLeaderStyle.Hyphens));
tabStops.Add(new NTabStop(500, ENTabStopAlignment.Decimal, ENTabStopLeaderStyle.Underline));
paragraph.TabStops = tabStops;
|
This results in the following paragraph:

A line break is represented by the NLineBreakInline element. It represents a formatting instruction for the paragraph and prohibits the appearance of other inlines after the break on the same line. The following code shows how to create a paragraph that contains a hard line break:
Changing the Tab Leader Style |
Copy Code
|
NParagraph paragraph = new NParagraph();
paragraph.Inlines.Add(new NTextInline("First line"));
paragraph.Inlines.Add(new NLineBreakInline());
paragraph.Inlines.Add(new NTextInline("Second line"));
|
A page break is represented by the NPageBreakInline element. It represents a formatting instruction in case the control uses a paging view. The following code snippet shows how to create a paragraph that contains an explicit page break:
Changing the Tab Leader Style |
Copy Code
|
NParagraph paragraph = new NParagraph();
paragraph.Inlines.Add(new NTextInline("First Page"));
paragraph.Inlines.Add(new NPageBreakInline());
paragraph.Inlines.Add(new NTextInline("Second Page"));
|
You can use the block level properties PageBreakBefore and PageBreakAfter to insert page breaks before or after the paragraph.
When the control uses paging, some paragraphs may end up with only a single line on a page or column. A paragraph ending line that falls at the beginning of the next page or column is called a widow, while a paragraph's first line that appears by itself at the bottom of a page or column is called an orphan. Widow and orphan lines generally decrease the readability of a paginated document. By default, the control will not allow widow and orphan lines in paragraphs when in paged view. However, you can disable this by setting the WidowOrphanControl property of the paragraph to false.
Changing the Tab Leader Style |
Copy Code
|
paragraph.WidowOrphanControl = false;
|
You can use the block level property AvoidPageBreaksInside to prevent a paragraph from spanning two pages/columns.