Tree maps visually represent hierarchical data through nested rectangles, with each branch of the tree assigned a rectangle that is subdivided into smaller rectangles representing sub-branches. The size of each node's rectangle corresponds to its value relative to the total value of all nodes at the same level in the tree. Optionally the color of the rectangle can represent the rate of change of the value of tree leaf or branch node. This combination of layout and color-coded information enables the user to identify significant branches or nodes within the data. Another advantage of tree maps is that, by construction, they make efficient use of space. As a result, they can legibly display large amounts of items on the screen. The following image shows a typical treemap chart:
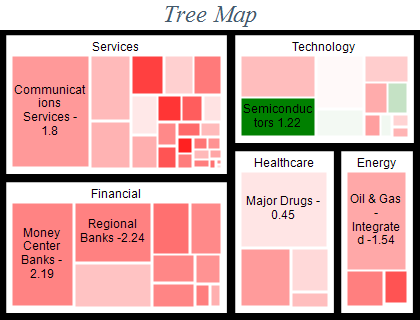
You create a Treemap chart by creating an instance of the NTreeMapChart class. There are two ways to achieve this - using a predefined chart type or doing it through code. The following code snippets show how each of those approaches works:
Creating a TreeMap Chart Using a Predefined Chart Type
C# |
Copy Code
|
chartView.Surface.CreatePredefinedChart(ENPredefinedChartType.TreeMap);
NTreeMapChart treeMap = (NTreeMapChart)chartView.Surface.Charts[0];
|
Creating a TreeMap Chart from Code
C# |
Copy Code
|
// create a dock panel
NDockPanel dockPanel = new NDockPanel();
chartView.Surface.Content = dockPanel;
// create a docked label
NLabel label = new NLabel();
label.Text = "TreeMap";
NDockLayout.SetDockArea(label, ENDockArea.Top);
dockPanel.AddChild(label);
// create a new legend
NLegend legend = new NLegend();
NDockLayout.SetDockArea(legend, ENDockArea.Right);
dockPanel.AddChild(legend);
// create a new funnel chart
NTreeMapChart treeMap = new NTreeMapChart();
NDockLayout.SetDockArea(treeMap, ENDockArea.Center);
dockPanel.AddChild(treeMap);
|
The data in the treemap is organized as a tree structure. The tree is created by adding instances of the NGroupTreeMapNode and NValueTreeMapNode classes that represent a branch and leaf (value) nodes respectively. The following code shows how to create the simple treemap:
C# |
Copy Code
|
// add some group and value nodes
NGroupTreeMapNode rootTreeMapNode = new NGroupTreeMapNode();
treeMap.RootTreeMapNode = rootTreeMapNode;
NGroupTreeMapNode technologyNode = new NGroupTreeMapNode("Technology");
technologyNode.Margins = new NMargins(2);
technologyNode.Border = NBorder.CreateFilledBorder(NColor.Black);
technologyNode.BorderThickness = new NMargins(2);
rootTreeMapNode.ChildNodes.Add(technologyNode);
technologyNode.ChildNodes.Add(new NValueTreeMapNode(656.4, -1.16, "Software Programming", new NColorFill(NColor.FromRGB(129, 200, 0))));
technologyNode.ChildNodes.Add(new NValueTreeMapNode(619.9, 1.22, "Semiconductors", new NColorFill(NColor.FromRGB(255, 204, 0))));
technologyNode.ChildNodes.Add(new NValueTreeMapNode(535.9, -0.1, "Communications Equipment", new NColorFill(NColor.FromRGB(241, 85, 0))));
technologyNode.ChildNodes.Add(new NValueTreeMapNode(277.1, 0.06, "Computer Hardware", new NColorFill(NColor.FromRGB(126, 0, 255))));
NGroupTreeMapNode healthcareNode = new NGroupTreeMapNode("Healthcare");
healthcareNode.Margins = new NMargins(2);
healthcareNode.Border = NBorder.CreateFilledBorder(NColor.Black);
healthcareNode.BorderThickness = new NMargins(2);
rootTreeMapNode.ChildNodes.Add(healthcareNode);
healthcareNode.ChildNodes.Add(new NValueTreeMapNode(446, -0.45, "Major Drugs", new NColorFill(NColor.FromRGB(129, 200, 0))));
healthcareNode.ChildNodes.Add(new NValueTreeMapNode(593.3, -1.77, "Biotechnology Drugs", new NColorFill(NColor.FromRGB(255, 204, 0))));
healthcareNode.ChildNodes.Add(new NValueTreeMapNode(325.4, -0.67, "Medical Equipment Supplies", new NColorFill(NColor.FromRGB(241, 85, 0))));
|
Each tree map node has a property called BackgroundFillStyle that allows you to assign explicit fill to the node. If you do not assign a fill the node the treemap will automatically assign one using the following rules:
1. If this is a value node then it will get the first available palette object from the ancestor chain of group nodes and assign a color that represents the value node's change value on that palette.
2. If this is a group node then it does not assign a fill.
To illustrate how this works consider that we don't specify an explicit color to the nodes and assign a palette to the root node:
C# |
Copy Code
|
//...
technologyNode.ChildNodes.Add(new NValueTreeMapNode(656.4, -1.16, "Software Programming"));
technologyNode.ChildNodes.Add(new NValueTreeMapNode(619.9, 1.22, "Semiconductors"));
technologyNode.ChildNodes.Add(new NValueTreeMapNode(535.9, -0.1, "Communications Equipment"));
//...
NThreeColorPalette palette = new NThreeColorPalette();
palette.BeginColor = NColor.Red;
palette.OriginColor = NColor.White;
palette.OriginValue = 0;
palette.EndColor = NColor.Green;
rootTreeMapNode.Palette = palette;
|
Then the tree map will automatically compute a color for the node based on the palette properties assigned to the root tree map node. The nodes with a bigger positive change get colored in more intense shades of green, whereas the nodes with a more negative change get colored in more intense shades of red. The palette settings are controlled from the palette object associated with the node parent group node. The following code shows how to create a custom palette:
C# |
Copy Code
|
NColorValuePalette palette = new NColorValuePalette();
palette.ColorValuePairs = new NDomArray<NColorValuePair>(new NColorValuePair[] {
new NColorValuePair(-2, NColor.Blue),
new NColorValuePair(0, NColor.White),
new NColorValuePair(2, NColor.Green) });
rootTreeMapNode.Palette = palette;
|
The NTreeMapNode (base class for NGroupTreeMapNode and NValueTreeMapNode) has a property called Format that defines the text that appears inside the tree map node rectangle. By default this property is set to "<label> <change_percent>" for value nodes and "<label>" for group nodes, meaning that value nodes by default will display the node label followed by the formatted change value and group nodes will display the node label only. The following table shows the different formatting commands you can use in the Format property:
Format Command |
Description |
label |
Applies to both value and group nodes. Replaces the command with the value of the Label property associated with the node. |
change |
Applies to value nodes only. Replaces the command with the formatted value of the Change property associated with the node. |
change_percent |
Applies to value nodes only. Replaces the command with the formatted percentage value of the Change property associated with the node. |
min_value |
Applies to group nodes only. Replaces the command with the formatted minimum value of its child nodes. |
max_value |
Applies to group nodes only. Replaces the command with the formatted maximum values of its child nodes. |
total_value |
Applies to group nodes only. Replaces the command with the formatted total sum of its child node's values. |
min_change |
Applies to group nodes only. Replaces the command with the formatted minimum change value of its child nodes. |
max_change |
Applies to group nodes only. Replaces the command with the formatted maximum change value of its child nodes. |
min_change_percent |
Applies to group nodes only. Replaces the command with the formatted minimum percentage change value of its child nodes. |
max_change_percent |
Applies to group nodes only. Replaces the command with the formatted maximum percentage change of its child nodes. |
The following example shows how to apply a custom format to the technology group and change the way change values are formatted to triple decimal precision:
C# |
Copy Code
|
technologyNode.Format = "<label> change from <min_change> to <max_change>";
technologyNode.ValueFormatter = new NNumericValueFormatter("0.000");
|
The text visualization properties of the tree map nodes such as font, style, font size, etc. are controlled from the le LabelTextStyle property of the node.
Each group tree map node can output information about itself or its descendants on a legend. This is controlled from the LegendView property of the group tree map node. The following code shows how to create a tree map with an associated legend:
C# |
Copy Code
|
// create a dock panel
NDockPanel dockPanel = new NDockPanel();
chartView.Surface.Content = dockPanel;
// create a docked label
NLabel label = new NLabel();
label.Text = "TreeMap";
NDockLayout.SetDockArea(label, ENDockArea.Top);
dockPanel.AddChild(label);
// create a new legend
NLegend legend = new NLegend();
NDockLayout.SetDockArea(legend, ENDockArea.Right);
dockPanel.AddChild(legend);
// create a new funnel chart
NTreeMapChart treeMap = new NTreeMapChart();
NDockLayout.SetDockArea(treeMap, ENDockArea.Center);
dockPanel.AddChild(treeMap);
// link the tree map to the legend
treeMap.Legend = legend;
// create a root node with an associated legend view
NGroupTreeMapNode rootTreeMapNode = new NGroupTreeMapNode();
NGroupTreeMapNodeLegendView legendView = new NGroupTreeMapNodeLegendView();
rootTreeMapNode.LegendView = legendView;
treeMap.RootTreeMapNode = rootTreeMapNode;
|
The NGroupTreeMapLegendView object is similar to the series legend view object except for the Mode property which accepts values from the ENTreeMapNodeLegendMode enumeration:
ENTreeMapNodeLegendMode |
Description |
None |
The node is not represented on the legend. |
Group |
The node is represented on the legend by one entry. |
ValueNodes |
The group node adds its child nodes to the legend. |
GroupAndChildNodes |
Both group and value nodes are exported to the legend. |
Palette |
The group node adds a palette to the legend. |
Each tree node has properties called Margins, Padding, BorderThickness, and Border that control the box generated by the node. The Margins property controls the left, top, right and bottom spacing applied to the box before the border is drawn. The Padding property controls the left, top, right, and bottom spacing of the box content area from the border (stroke). The following code snippets show how to apply margins, border, and padding:
C# |
Copy Code
|
treeMapNode.Margins = new NMargins(2);
treeMapNode.Border = NBorder.CreateFilledBorder(NColor.Black);
treeMapNode.BorderThickness = new NMargins(2);
treeMapNode.Padding = new NMargins(1);
|
TreeMap Node Label Fit Modes
The treemap node has a property called LabelFitModes that controls how the label inside the box is fitted inside the node content area. The following table lists the options of the ENTreeMapNodeLabelFitMode enumeration:
ENTreeMapNodeLabelFitMode |
Description |
Hide |
Automatically hides the label if its size exceeds the size of the node. Always succeeds. |
Wrap |
Wraps the label if its size exceeds the size of the node. Always succeeds. |
Trim |
Trims the label if its size exceeds the size of the node. Always succeeds. |
AutoScale |
Automatically scales the label so that it fits the bounds of the node. Will succeed if the computed scale factor is bigger than MinAutoScale. |
You can specify one or more label fit modes as shown in the following example:
C# |
Copy Code
|
treeMapNode.LabelFitModes = new NDomArray<ENTreeMapNodeLabelFitMode>(new ENTreeMapNodeLabelFitMode[] { ENTreeMapNodeLabelFitMode.AutoScale, ENTreeMapNodeLabelFitMode.Hide });
treeMapNode.MinAutoScale = 0.5;
|
The above code will instruct the node to auto scale labels that don't fit. If the computed auto scaling factor is below 0.5 (e.g. the font size needed to display the label drops more than half) the label will be hidden.